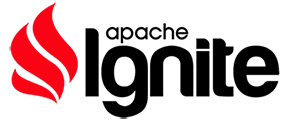 |
Apache Ignite C++
|
Go to the documentation of this file.
23 #ifndef _IGNITE_IGNITE
24 #define _IGNITE_IGNITE
26 #include <ignite/impl/ignite_impl.h>
47 friend class impl::IgniteImpl;
57 Ignite(impl::IgniteImpl* impl);
84 const char* GetName()
const;
103 template<
typename K,
typename V>
124 template<
typename K,
typename V>
127 impl::cache::CacheImpl* cacheImpl = impl.Get()->GetCache(name, err);
140 template<
typename K,
typename V>
161 template<
typename K,
typename V>
164 impl::cache::CacheImpl* cacheImpl = impl.Get()->GetOrCreateCache(name, err);
177 template<
typename K,
typename V>
198 template<
typename K,
typename V>
201 impl::cache::CacheImpl* cacheImpl = impl.Get()->CreateCache(name, err);
219 void SetActive(
bool active);
282 return impl.IsValid();
287 ignite::common::concurrent::SharedPointer<impl::IgniteImpl> impl;
291 #endif //_IGNITE_IGNITE
Ignite Binding.
Definition: ignite_binding.h:38
Defines compute grid functionality for executing tasks and closures over nodes in the ClusterGroup.
Definition: compute.h:74
Transactions facade.
Definition: core/include/ignite/transactions/transactions.h:45
Apache Ignite API.
Definition: cache.h:48
bool IsValid() const
Check if the instance is valid.
Definition: ignite.h:280
cache::Cache< K, V > CreateCache(const char *name, IgniteError &err)
Create cache.
Definition: ignite.h:199
Main entry point for all Data Grid APIs.
Definition: cache.h:68
cache::CacheAffinity< K > GetAffinity(const std::string &cacheName)
Get affinity service to provide information about data partitioning and distribution.
Definition: ignite.h:68
Main interface to operate with Ignite.
Definition: ignite.h:45
Defines a cluster group which contains all or a subset of cluster nodes.
Definition: cluster_group.h:45
Provides affinity information to detect which node is primary and which nodes are backups for a parti...
Definition: cache_affinity.h:42
cache::Cache< K, V > GetOrCreateCache(const char *name, IgniteError &err)
Get or create cache.
Definition: ignite.h:162
cache::Cache< K, V > GetCache(const char *name, IgniteError &err)
Get cache.
Definition: ignite.h:125
cache::Cache< K, V > CreateCache(const char *name)
Create cache.
Definition: ignite.h:178
Ignite configuration.
Definition: ignite_configuration.h:35
static void ThrowIfNeeded(const IgniteError &err)
Throw an error if code is not IGNITE_SUCCESS.
Definition: ignite_error.cpp:27
Represents whole cluster (all available nodes).
Definition: ignite_cluster.h:39
Ignite error information.
Definition: ignite_error.h:94
cache::Cache< K, V > GetCache(const char *name)
Get cache.
Definition: ignite.h:104
cache::Cache< K, V > GetOrCreateCache(const char *name)
Get or create cache.
Definition: ignite.h:141