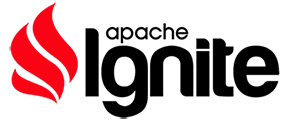 |
Apache Ignite C++
|
Go to the documentation of this file.
23 #ifndef _IGNITE_BINARY_BINARY_RAW_READER
24 #define _IGNITE_BINARY_BINARY_RAW_READER
29 #include <ignite/common/common.h>
31 #include "ignite/impl/binary/binary_reader_impl.h"
86 int32_t ReadInt8Array(int8_t* res, int32_t len);
105 int32_t ReadBoolArray(
bool* res, int32_t len);
124 int32_t ReadInt16Array(int16_t* res, int32_t len);
131 uint16_t ReadUInt16();
143 int32_t ReadUInt16Array(uint16_t* res, int32_t len);
162 int32_t ReadInt32Array(int32_t* res, int32_t len);
181 int32_t ReadInt64Array(int64_t* res, int32_t len);
200 int32_t ReadFloatArray(
float* res, int32_t len);
219 int32_t ReadDoubleArray(
double* res, int32_t len);
238 int32_t ReadGuidArray(
Guid* res, int32_t len);
257 int32_t ReadDateArray(
Date* res, int32_t len);
276 int32_t ReadTimestampArray(
Timestamp* res, int32_t len);
295 int32_t ReadTimeArray(
Time* res, int32_t len);
308 int32_t ReadString(
char* res, int32_t len);
331 int32_t len = ReadString(NULL, 0);
335 dst.resize(
static_cast<size_t>(len));
337 ReadString(&dst[0], len);
377 int32_t
id = impl->ReadArray(&size);
398 int32_t
id = impl->ReadCollection(&typ, &size);
409 template<
typename T,
typename OutputIterator>
412 return impl->ReadCollection<T>(out);
425 template<
typename K,
typename V>
431 int32_t
id = impl->ReadMap(&typ, &size);
448 int32_t ReadCollectionSize();
460 return impl->ReadObject<T>();
473 return impl->ReadEnum<T>();
488 if (impl->SkipIfNull())
491 res = impl->ReadObject<T>();
498 ignite::impl::binary::BinaryReaderImpl* impl;
503 #endif //_IGNITE_BINARY_BINARY_RAW_READER
Binary raw reader.
Definition: binary_raw_reader.h:57
Apache Ignite API.
Definition: cache.h:48
Type
Definition: binary_consts.h:69
Time type.
Definition: time.h:35
BinaryMapReader< K, V > ReadMap()
Start map read.
Definition: binary_raw_reader.h:426
Global universally unique identifier (GUID).
Definition: guid.h:36
Binary collection reader.
Definition: binary_containers.h:468
Binary map reader.
Definition: binary_containers.h:561
int32_t ReadCollection(OutputIterator out)
Read values and insert them to specified position.
Definition: binary_raw_reader.h:410
BinaryCollectionReader< T > ReadCollection()
Start collection read.
Definition: binary_raw_reader.h:393
bool TryReadObject(T &res)
Try read object.
Definition: binary_raw_reader.h:486
T ReadObject()
Read object.
Definition: binary_raw_reader.h:458
BinaryArrayReader< T > ReadArray()
Start array read.
Definition: binary_raw_reader.h:373
Binary array reader.
Definition: binary_containers.h:389
Binary enum entry.
Definition: binary_enum_entry.h:39
Timestamp type.
Definition: timestamp.h:37
Date type.
Definition: date.h:35
Binary string array reader.
Definition: binary_containers.h:297
std::string ReadString()
Read string from the stream.
Definition: binary_raw_reader.h:315
Type
Definition: binary_consts.h:35
T ReadEnum()
Read enum value.
Definition: binary_raw_reader.h:471
void ReadString(std::string &dst)
Read string from the stream.
Definition: binary_raw_reader.h:329