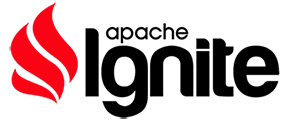 |
Apache Ignite C++
|
Go to the documentation of this file.
23 #ifndef _IGNITE_BINARY_BINARY_CONTAINERS
24 #define _IGNITE_BINARY_BINARY_CONTAINERS
28 #include <ignite/common/utils.h>
30 #include "ignite/impl/binary/binary_writer_impl.h"
31 #include "ignite/impl/binary/binary_reader_impl.h"
67 void Write(
const char* val);
77 void Write(
const char* val, int32_t len);
86 void Write(
const std::string& val)
103 impl::binary::BinaryWriterImpl* impl;
146 impl->WriteElement<T>(id, val);
159 impl->CommitContainer(
id);
164 impl::binary::BinaryWriterImpl* impl;
207 impl->WriteElement<T>(id, val);
220 impl->CommitContainer(
id);
224 impl::binary::BinaryWriterImpl* impl;
240 template<
typename K,
typename V>
265 void Write(
const K& key,
const V& val)
267 impl->WriteElement<K, V>(id, key, val);
279 impl->CommitContainer(
id);
283 impl::binary::BinaryWriterImpl* impl;
330 int32_t GetNext(
char* res, int32_t len);
341 int32_t len = GetNext(NULL, 0);
345 ignite::common::FixedSizeArray<char> arr(len + 1);
347 GetNext(arr.GetData(),
static_cast<int32_t
>(arr.GetSize()));
349 return std::string(arr.GetData());
352 return std::string();
360 int32_t GetSize()
const;
371 impl::binary::BinaryReaderImpl* impl;
401 impl(impl), id(id), size(size)
413 return impl->HasNextElement(
id);
425 return impl->ReadElement<T>(id);
449 impl::binary::BinaryReaderImpl* impl;
493 return impl->HasNextElement(
id);
505 return impl->ReadElement<T>(id);
540 impl::binary::BinaryReaderImpl* impl;
560 template<
typename K,
typename V>
574 int32_t size) : impl(impl), id(id), type(type), size(size)
586 return impl->HasNextElement(
id);
601 return impl->ReadElement<K, V>(id, key, val);
636 impl::binary::BinaryReaderImpl* impl;
650 #endif //_IGNITE_BINARY_BINARY_CONTAINERS
void Close()
Close the writer.
Definition: binary_containers.h:218
Apache Ignite API.
Definition: cache.h:48
BinaryMapWriter(impl::binary::BinaryWriterImpl *impl, int32_t id)
Constructor.
Definition: binary_containers.h:251
Binary string array writer.
Definition: binary_containers.h:48
Type
Definition: binary_consts.h:69
void Write(const T &val)
Write a value.
Definition: binary_containers.h:205
void Write(const std::string &val)
Write string.
Definition: binary_containers.h:86
BinaryCollectionReader(impl::binary::BinaryReaderImpl *impl, int32_t id, const CollectionType::Type type, int32_t size)
Constructor.
Definition: binary_containers.h:480
BinaryArrayWriter(impl::binary::BinaryWriterImpl *impl, int32_t id)
Constructor.
Definition: binary_containers.h:131
Binary collection reader.
Definition: binary_containers.h:468
T GetNext()
Read next element.
Definition: binary_containers.h:423
int32_t GetSize()
Get collection size.
Definition: binary_containers.h:524
Binary map reader.
Definition: binary_containers.h:561
void Close()
Close the writer.
Definition: binary_containers.h:277
bool IsNull()
Check whether collection is NULL.
Definition: binary_containers.h:534
BinaryCollectionWriter(impl::binary::BinaryWriterImpl *impl, int32_t id)
Constructor.
Definition: binary_containers.h:192
void Close()
Close the writer.
Definition: binary_containers.h:157
int32_t GetSize()
Get array size.
Definition: binary_containers.h:433
Binary array writer.
Definition: binary_containers.h:121
bool IsNull()
Check whether array is NULL.
Definition: binary_containers.h:443
Binary array reader.
Definition: binary_containers.h:389
bool HasNext()
Check whether next element is available for read.
Definition: binary_containers.h:584
Binary collection writer.
Definition: binary_containers.h:182
void Write(const T &val)
Write a value.
Definition: binary_containers.h:144
bool IsNull()
Check whether map is NULL.
Definition: binary_containers.h:630
Binary string array reader.
Definition: binary_containers.h:297
Binary map writer.
Definition: binary_containers.h:241
Type
Definition: binary_consts.h:35
bool HasNext()
Check whether next element is available for read.
Definition: binary_containers.h:491
BinaryArrayReader(impl::binary::BinaryReaderImpl *impl, int32_t id, int32_t size)
Constructor.
Definition: binary_containers.h:400
std::string GetNext()
Get next element.
Definition: binary_containers.h:339
T GetNext()
Read next element.
Definition: binary_containers.h:503
BinaryMapReader(impl::binary::BinaryReaderImpl *impl, int32_t id, MapType::Type type, int32_t size)
Constructor.
Definition: binary_containers.h:573
void GetNext(K &key, V &val)
Read next element.
Definition: binary_containers.h:599
bool HasNext()
Check whether next element is available for read.
Definition: binary_containers.h:411
int32_t GetSize()
Get map size.
Definition: binary_containers.h:620
MapType::Type GetType()
Get map type.
Definition: binary_containers.h:610
void Write(const K &key, const V &val)
Write a map entry.
Definition: binary_containers.h:265
CollectionType::Type GetType()
Get collection type.
Definition: binary_containers.h:514