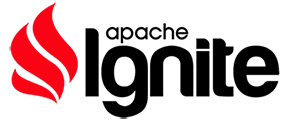 |
Apache Ignite C++
|
Go to the documentation of this file.
23 #ifndef _IGNITE_THIN_CACHE_QUERY_QUERY_SQL_FIELDS
24 #define _IGNITE_THIN_CACHE_QUERY_QUERY_SQL_FIELDS
30 #include <ignite/impl/thin/copyable_writable.h>
39 class SqlFieldsQueryRequest;
55 friend class ignite::impl::thin::SqlFieldsQueryRequest;
69 distributedJoins(false),
70 enforceJoinOrder(false),
88 pageSize(other.pageSize),
89 maxRows(other.maxRows),
90 timeout(other.timeout),
92 distributedJoins(other.distributedJoins),
93 enforceJoinOrder(other.enforceJoinOrder),
95 collocated(other.collocated),
97 updateBatchSize(other.updateBatchSize),
100 args.reserve(other.args.size());
102 typedef std::vector<impl::thin::CopyableWritable*>::const_iterator Iter;
104 for (Iter i = other.args.begin(); i != other.args.end(); ++i)
105 args.push_back((*i)->Copy());
144 swap(sql, other.sql);
145 swap(schema, other.schema);
146 swap(pageSize, other.pageSize);
147 swap(maxRows, other.maxRows);
148 swap(timeout, other.timeout);
149 swap(loc, other.loc);
150 swap(distributedJoins, other.distributedJoins);
151 swap(enforceJoinOrder, other.enforceJoinOrder);
152 swap(lazy, other.lazy);
153 swap(collocated, other.collocated);
154 swap(parts, other.parts);
155 swap(args, other.args);
188 this->schema = schema;
221 this->pageSize = pageSize;
231 this->maxRows = maxRows;
251 this->timeout = timeout;
295 return distributedJoins;
308 distributedJoins = enabled;
318 return enforceJoinOrder;
334 enforceJoinOrder = enforce;
392 this->collocated = collocated;
416 this->parts = partitions;
426 updateBatchSize = size;
436 return updateBatchSize;
450 args.push_back(
new impl::thin::CopyableWritableImpl<T>(arg));
461 template<
typename Iter>
464 args.push_back(
new impl::thin::CopyableWritableInt8ArrayImpl<Iter>(begin, end));
472 std::vector<impl::thin::CopyableWritable*>::iterator iter;
473 for (iter = args.begin(); iter != args.end(); ++iter)
499 bool distributedJoins;
502 bool enforceJoinOrder;
511 std::vector<int32_t> parts;
514 int32_t updateBatchSize;
517 std::vector<impl::thin::CopyableWritable*> args;
524 #endif //_IGNITE_THIN_CACHE_QUERY_QUERY_SQL_FIELDS
void Swap(SqlFieldsQuery &other)
Efficiently swaps contents with another SqlQuery instance.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:138
SqlFieldsQuery(const SqlFieldsQuery &other)
Copy constructor.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:85
bool IsCollocated()
Checks if this query is collocated.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:374
Apache Ignite API.
Definition: cache.h:48
void SetMaxRows(int32_t maxRows)
Set maximum number of rows.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:229
int64_t GetTimeout() const
Get query execution timeout in milliseconds.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:259
void SetUpdateBatchSize(int32_t size)
Set batch size for update queries.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:424
bool IsLazy() const
Gets lazy query execution flag.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:344
SqlFieldsQuery(const std::string &sql)
Constructor.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:62
void SetSql(const std::string &sql)
Set SQL string.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:174
~SqlFieldsQuery()
Destructor.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:128
void SetCollocated(bool collocated)
Sets flag defining if this query is collocated.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:390
SQL fields query for thin client.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:52
void SetLocal(bool loc)
Set local flag.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:283
const std::string & GetSql() const
Get SQL string.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:164
SqlFieldsQuery & operator=(const SqlFieldsQuery &other)
Assignment operator.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:113
void AddInt8ArrayArgument(Iter begin, Iter end)
Add int8_t array as an argument.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:462
void ClearArguments()
Remove all added arguments.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:470
int32_t GetUpdateBatchSize() const
Get batch size for update queries.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:434
bool IsEnforceJoinOrder() const
Checks if join order of tables if enforced.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:316
bool IsLocal() const
Get local flag.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:271
void SetPartitions(const std::vector< int32_t > &partitions)
Set partitions for the query.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:414
void SetEnforceJoinOrder(bool enforce)
Sets flag to enforce join order of tables in the query.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:332
void SetDistributedJoins(bool enabled)
Specify if distributed joins are enabled for this query.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:306
void SetSchema(const std::string &schema)
Set schema name for the query.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:186
void AddArgument(const T &arg)
Add argument for the query.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:448
void SetPageSize(int32_t pageSize)
Set page size.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:219
int32_t GetMaxRows() const
Get maximum number of rows.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:239
int32_t GetPageSize() const
Get page size.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:209
void SetLazy(bool lazy)
Sets lazy query execution flag.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:364
void SetTimeout(int64_t timeout)
Set query execution timeout in milliseconds.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:249
const std::string & GetSchema() const
Get schema name for the query.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:199
const std::vector< int32_t > & GetPartitions() const
Get partitions for the query.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:402
bool IsDistributedJoins() const
Check if distributed joins are enabled for this query.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:293