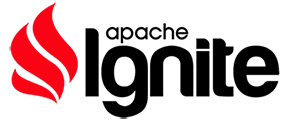 |
Apache Ignite C++
|
Go to the documentation of this file.
23 #ifndef _IGNITE_THIN_CACHE_QUERY_QUERY_SCAN
24 #define _IGNITE_THIN_CACHE_QUERY_QUERY_SCAN
30 #include <ignite/impl/thin/copyable_writable.h>
39 class ScanQueryRequest;
55 friend class ScanQueryRequest;
82 this->part = partition;
102 this->pageSize = resultPageSize;
144 #endif //_IGNITE_THIN_CACHE_QUERY_QUERY_SCAN
Apache Ignite API.
Definition: cache.h:48
int32_t GetPartition() const
Get partition to scan.
Definition: thin-client/include/ignite/thin/cache/query/query_scan.h:70
void SetLocal(bool localScan)
Set local flag.
Definition: thin-client/include/ignite/thin/cache/query/query_scan.h:124
Scan query.
Definition: thin-client/include/ignite/thin/cache/query/query_scan.h:52
ScanQuery()
Default constructor.
Definition: thin-client/include/ignite/thin/cache/query/query_scan.h:60
void SetPartition(int32_t partition)
Set partition to scan.
Definition: thin-client/include/ignite/thin/cache/query/query_scan.h:80
int32_t GetPageSize() const
Get page size.
Definition: thin-client/include/ignite/thin/cache/query/query_scan.h:90
void SetPageSize(int32_t resultPageSize)
Set the size of the result page.
Definition: thin-client/include/ignite/thin/cache/query/query_scan.h:100
bool IsLocal() const
Get local flag.
Definition: thin-client/include/ignite/thin/cache/query/query_scan.h:112