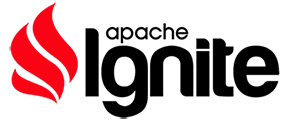 |
Apache Ignite C++
|
Go to the documentation of this file.
23 #ifndef _IGNITE_THIN_CACHE_EVENT_CACHE_ENTRY_EVENT
24 #define _IGNITE_THIN_CACHE_EVENT_CACHE_ENTRY_EVENT
41 template<
typename K,
typename V>
72 static Type FromInt8(int8_t val)
80 return static_cast<Type>(val);
85 "Unsupported CacheEntryEventType",
"val", val);
97 template<
typename K,
typename V>
124 oldVal(other.oldVal),
125 hasOldValue(other.hasOldValue),
126 eventType(other.eventType)
151 oldVal = other.oldVal;
152 hasOldValue = other.hasOldValue;
153 eventType = other.eventType;
204 int8_t eventTypeByte = reader.
ReadInt8();
205 this->eventType = CacheEntryEventType::FromInt8(eventTypeByte);
226 #endif //_IGNITE_THIN_CACHE_EVENT_CACHE_ENTRY_EVENT
Cache entry event class template.
Definition: thin-client/include/ignite/thin/cache/event/cache_entry_event.h:98
@ CREATED
An event type indicating that the cache entry was created.
Definition: thin-client/include/ignite/thin/cache/event/cache_entry_event.h:60
Binary raw reader.
Definition: binary_raw_reader.h:57
Apache Ignite API.
Definition: cache.h:48
CacheEntry & operator=(const CacheEntry &other)
Assignment operator.
Definition: thin-client/include/ignite/thin/cache/cache_entry.h:121
Cache entry class template.
Definition: thin-client/include/ignite/thin/cache/cache_entry.h:50
bool hasValue
Indicates whether value exists.
Definition: thin-client/include/ignite/thin/cache/cache_entry.h:171
@ EXPIRED
An event type indicating that the cache entry was removed by expiration policy.
Definition: thin-client/include/ignite/thin/cache/event/cache_entry_event.h:69
@ REMOVED
An event type indicating that the cache entry was removed.
Definition: thin-client/include/ignite/thin/cache/event/cache_entry_event.h:66
Definition: thin-client/include/ignite/thin/cache/event/cache_entry_event.h:42
@ UPDATED
An event type indicating that the cache entry was updated.
Definition: thin-client/include/ignite/thin/cache/event/cache_entry_event.h:63
virtual ~CacheEntryEvent()
Destructor.
Definition: thin-client/include/ignite/thin/cache/event/cache_entry_event.h:134
CacheEntryEvent(const CacheEntryEvent< K, V > &other)
Copy constructor.
Definition: thin-client/include/ignite/thin/cache/event/cache_entry_event.h:122
bool TryReadObject(T &res)
Try read object.
Definition: binary_raw_reader.h:486
K key
Key.
Definition: thin-client/include/ignite/thin/cache/cache_entry.h:165
V val
Value.
Definition: thin-client/include/ignite/thin/cache/cache_entry.h:168
T ReadObject()
Read object.
Definition: binary_raw_reader.h:458
Type
Definition: thin-client/include/ignite/thin/cache/event/cache_entry_event.h:57
CacheEntryEvent()
Default constructor.
Definition: thin-client/include/ignite/thin/cache/event/cache_entry_event.h:108
Cache event type.
Definition: thin-client/include/ignite/thin/cache/event/cache_entry_event.h:55
const V & GetOldValue() const
Get old value.
Definition: thin-client/include/ignite/thin/cache/event/cache_entry_event.h:176
bool HasOldValue() const
Check if the old value exists.
Definition: thin-client/include/ignite/thin/cache/event/cache_entry_event.h:186
int8_t ReadInt8()
Read 8-byte signed integer.
Definition: binary_raw_reader.cpp:32
static const int IGNITE_ERR_BINARY
Binary error.
Definition: ignite_error.h:125
CacheEntryEventType::Type GetEventType() const
Get event type.
Definition: thin-client/include/ignite/thin/cache/event/cache_entry_event.h:166
CacheEntryEvent & operator=(const CacheEntryEvent< K, V > &other)
Assignment operator.
Definition: thin-client/include/ignite/thin/cache/event/cache_entry_event.h:145