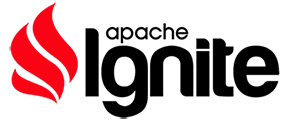 |
Apache Ignite C++
|
Go to the documentation of this file.
23 #ifndef _IGNITE_COMMON_REFERENCE
24 #define _IGNITE_COMMON_REFERENCE
28 #include <ignite/common/common.h>
29 #include <ignite/common/concurrent.h>
30 #include <ignite/common/reference_impl.h>
72 explicit ConstReference(common::ConstReferenceImplBase* ptr, ptrdiff_t offset = 0) :
104 T2* p0 =
reinterpret_cast<T2*
>(common::POINTER_CAST_MAGIC_NUMBER);
105 T* p1 =
static_cast<T*
>(p0);
107 ptrdiff_t diff =
reinterpret_cast<ptrdiff_t
>(p1) -
reinterpret_cast<ptrdiff_t
>(p0);
119 offset = other.offset;
132 template<
typename T2>
136 offset = other.offset;
138 T2* p0 =
reinterpret_cast<T2*
>(common::POINTER_CAST_MAGIC_NUMBER);
139 T* p1 =
static_cast<T*
>(p0);
141 ptrdiff_t diff =
reinterpret_cast<ptrdiff_t
>(p1) -
reinterpret_cast<ptrdiff_t
>(p0);
165 return reinterpret_cast<const T*
>(
reinterpret_cast<ptrdiff_t
>(ptr.Get()->Get()) + offset);
175 const common::ConstReferenceImplBase* raw = ptr.Get();
177 return !raw || !raw->Get();
182 common::concurrent::SharedPointer<common::ConstReferenceImplBase> ptr;
200 friend class Reference;
218 explicit Reference(common::ReferenceImplBase* ptr, ptrdiff_t offset = 0) :
244 template<
typename T2>
249 T2* p0 =
reinterpret_cast<T2*
>(common::POINTER_CAST_MAGIC_NUMBER);
250 T* p1 =
static_cast<T*
>(p0);
252 ptrdiff_t diff =
reinterpret_cast<ptrdiff_t
>(p1) -
reinterpret_cast<ptrdiff_t
>(p0);
264 offset = other.offset;
276 template<
typename T2>
280 offset = other.offset;
282 T2* p0 =
reinterpret_cast<T2*
>(common::POINTER_CAST_MAGIC_NUMBER);
283 T* p1 =
static_cast<T*
>(p0);
285 ptrdiff_t diff =
reinterpret_cast<ptrdiff_t
>(p1) -
reinterpret_cast<ptrdiff_t
>(p0);
306 template<
typename T2>
314 T2* p0 =
reinterpret_cast<T2*
>(common::POINTER_CAST_MAGIC_NUMBER);
315 const T* p1 =
static_cast<T*
>(p0);
317 ptrdiff_t diff =
reinterpret_cast<ptrdiff_t
>(p1) -
reinterpret_cast<ptrdiff_t
>(p0);
333 return reinterpret_cast<const T*
>(
reinterpret_cast<ptrdiff_t
>(ptr.Get()->Get()) + offset);
346 return reinterpret_cast<T*
>(
reinterpret_cast<ptrdiff_t
>(ptr.Get()->Get()) + offset);
356 const common::ReferenceImplBase* raw = ptr.Get();
358 return !raw || !raw->Get();
363 common::concurrent::SharedPointer<common::ReferenceImplBase> ptr;
386 common::ReferenceSmartPointer<T>* impl =
new common::ReferenceSmartPointer<T>();
412 common::ReferenceSmartPointer<T>* impl =
new common::ReferenceSmartPointer<T>();
433 common::ReferenceOwningRawPointer<T>* impl =
new common::ReferenceOwningRawPointer<T>(
new T(val));
450 common::ReferenceOwningRawPointer<T>* impl =
new common::ReferenceOwningRawPointer<T>(
new T(val));
468 common::ReferenceOwningRawPointer<T>* impl =
new common::ReferenceOwningRawPointer<T>(val);
486 common::ReferenceOwningRawPointer<T>* impl =
new common::ReferenceOwningRawPointer<T>(val);
504 common::ReferenceNonOwningRawPointer<T>* impl =
new common::ReferenceNonOwningRawPointer<T>(&val);
522 common::ReferenceNonOwningRawPointer<T>* impl =
new common::ReferenceNonOwningRawPointer<T>(val);
540 common::ConstReferenceNonOwningRawPointer<T>* impl =
new common::ConstReferenceNonOwningRawPointer<T>(&val);
558 common::ConstReferenceNonOwningRawPointer<T>* impl =
new common::ConstReferenceNonOwningRawPointer<T>(val);
564 #endif //_IGNITE_COMMON_REFERENCE
ConstReference< T > MakeConstReferenceFromOwningPointer(T *val)
Make ignite::ConstReference instance out of pointer and pass its ownership.
Definition: reference.h:484
Apache Ignite API.
Definition: cache.h:48
ConstReference(common::ConstReferenceImplBase *ptr, ptrdiff_t offset=0)
Constructor.
Definition: reference.h:72
~Reference()
Destructor.
Definition: reference.h:294
Reference< T > MakeReference(T &val)
Make ignite::Reference instance out of reference.
Definition: reference.h:502
ConstReference()
Default constructor.
Definition: reference.h:59
ConstReference< T > MakeConstReference(const T &val)
Make ignite::ConstReference instance out of constant reference.
Definition: reference.h:538
Reference< typename T::element_type > MakeReferenceFromSmartPointer(T ptr)
Make ignite::Reference instance out of smart pointer.
Definition: reference.h:384
ConstReference(const ConstReference &other)
Copy constructor.
Definition: reference.h:84
ConstReference & operator=(const ConstReference &other)
Assignment operator.
Definition: reference.h:116
Reference & operator=(const Reference< T2 > &other)
Assignment operator.
Definition: reference.h:277
Reference(const Reference &other)
Copy constructor.
Definition: reference.h:230
const T * Get() const
Dereference the pointer.
Definition: reference.h:331
Reference()
Default constructor.
Definition: reference.h:205
ConstReference< typename T::element_type > MakeConstReferenceFromSmartPointer(T ptr)
Make ignite::ConstReference instance out of smart pointer.
Definition: reference.h:410
Reference< T > MakeReferenceFromCopy(const T &val)
Copy object and wrap it to make ignite::Reference instance.
Definition: reference.h:431
T * Get()
Dereference the pointer.
Definition: reference.h:344
Reference(const Reference< T2 > &other)
Copy constructor.
Definition: reference.h:245
ConstReference< T > MakeConstReferenceFromCopy(const T &val)
Copy object and wrap it to make ignite::ConstReference instance.
Definition: reference.h:448
ConstReference & operator=(const ConstReference< T2 > &other)
Assignment operator.
Definition: reference.h:133
bool IsNull() const
Check if the pointer is null.
Definition: reference.h:173
const T * Get() const
Dereference the pointer.
Definition: reference.h:163
bool IsNull() const
Check if the pointer is null.
Definition: reference.h:354
~ConstReference()
Destructor.
Definition: reference.h:150
Reference class.
Definition: reference.h:35
Reference & operator=(const Reference &other)
Assignment operator.
Definition: reference.h:261
Reference< T > MakeReferenceFromOwningPointer(T *val)
Make ignite::Reference instance out of pointer and pass its ownership.
Definition: reference.h:466
Constant Reference class.
Definition: reference.h:47
ConstReference(const ConstReference< T2 > &other)
Copy constructor.
Definition: reference.h:100
Reference(common::ReferenceImplBase *ptr, ptrdiff_t offset=0)
Constructor.
Definition: reference.h:218