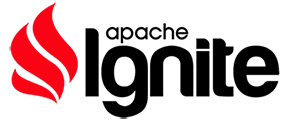 |
Apache Ignite C++
|
Go to the documentation of this file.
23 #ifndef _IGNITE_CACHE_QUERY_QUERY_SQL
24 #define _IGNITE_CACHE_QUERY_QUERY_SQL
30 #include <ignite/impl/writable_object.h>
53 SqlQuery(
const std::string& type,
const std::string& sql) :
58 distributedJoins(false),
72 pageSize(other.pageSize),
74 distributedJoins(other.distributedJoins),
77 args.reserve(other.args.size());
79 typedef std::vector<impl::WritableObjectBase*>::const_iterator Iter;
81 for (Iter i = other.args.begin(); i != other.args.end(); ++i)
82 args.push_back((*i)->Copy());
107 typedef std::vector<impl::WritableObjectBase*>::const_iterator Iter;
109 for (Iter it = args.begin(); it != args.end(); ++it)
122 std::swap(type, other.type);
123 std::swap(sql, other.sql);
124 std::swap(pageSize, other.pageSize);
125 std::swap(loc, other.loc);
126 std::swap(distributedJoins, other.distributedJoins);
127 std::swap(args, other.args);
188 this->pageSize = pageSize;
220 return distributedJoins;
233 distributedJoins = enabled;
248 args.push_back(
new impl::WritableObject<T>(arg));
256 std::vector<impl::WritableObjectBase*>::iterator iter;
257 for (iter = args.begin(); iter != args.end(); ++iter)
275 writer.
WriteInt32(
static_cast<int32_t
>(args.size()));
277 std::vector<impl::WritableObjectBase*>::const_iterator it;
279 for (it = args.begin(); it != args.end(); ++it)
280 (*it)->Write(writer);
301 bool distributedJoins;
304 std::vector<impl::WritableObjectBase*> args;
310 #endif //_IGNITE_CACHE_QUERY_QUERY_SQL
void SetType(const std::string &type)
Set type name.
Definition: query_sql.h:146
void SetPageSize(int32_t pageSize)
Set page size.
Definition: query_sql.h:186
Apache Ignite API.
Definition: cache.h:48
~SqlQuery()
Destructor.
Definition: query_sql.h:105
void WriteInt32(int32_t val)
Write 32-byte signed integer.
Definition: binary_raw_writer.cpp:72
const std::string & GetType() const
Get type name.
Definition: query_sql.h:136
SqlQuery & operator=(const SqlQuery &other)
Assignment operator.
Definition: query_sql.h:90
SqlQuery(const SqlQuery &other)
Copy constructor.
Definition: query_sql.h:69
int32_t GetPageSize() const
Get page size.
Definition: query_sql.h:176
void SetLocal(bool loc)
Set local flag.
Definition: query_sql.h:208
void AddArgument(const T &arg)
Add argument.
Definition: query_sql.h:246
Binary raw writer.
Definition: binary_raw_writer.h:62
const std::string & GetSql() const
Get SQL string.
Definition: query_sql.h:156
void SetSql(const std::string &sql)
Set SQL string.
Definition: query_sql.h:166
void Write(binary::BinaryRawWriter &writer) const
Write query info to the stream.
Definition: query_sql.h:268
void Swap(SqlQuery &other)
Efficiently swaps contents with another SqlQuery instance.
Definition: query_sql.h:118
void WriteString(const char *val)
Write string.
Definition: binary_raw_writer.cpp:152
bool IsLocal() const
Get local flag.
Definition: query_sql.h:196
void WriteBool(bool val)
Write bool.
Definition: binary_raw_writer.cpp:42
void ClearArguments()
Remove all added arguments.
Definition: query_sql.h:254
void SetDistributedJoins(bool enabled)
Specify if distributed joins are enabled for this query.
Definition: query_sql.h:231
bool IsDistributedJoins() const
Check if distributed joins are enabled for this query.
Definition: query_sql.h:218
SqlQuery(const std::string &type, const std::string &sql)
Constructor.
Definition: query_sql.h:53
Sql query.
Definition: query_sql.h:44