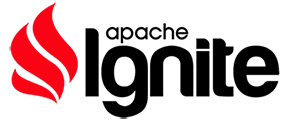 |
Apache Ignite C++
|
Go to the documentation of this file.
23 #ifndef _IGNITE_THIN_IGNITE_CLIENT
24 #define _IGNITE_THIN_IGNITE_CLIENT
28 #include <ignite/common/concurrent.h>
33 #include <ignite/thin/transactions/transactions.h>
51 typedef common::concurrent::SharedPointer<void> SP_Void;
81 template<
typename K,
typename V>
93 template<
typename K,
typename V>
105 template<
typename K,
typename V>
116 void DestroyCache(
const char* name);
124 void GetCacheNames(std::vector<std::string>& cacheNames);
150 SP_Void InternalGetCache(
const char* name);
159 SP_Void InternalGetOrCreateCache(
const char* name);
168 SP_Void InternalCreateCache(
const char* name);
176 SP_Void InternalTransactions();
184 SP_Void InternalCompute();
199 #endif // _IGNITE_THIN_IGNITE_CLIENT
cache::CacheClient< K, V > CreateCache(const char *name)
Create cache.
Definition: ignite_client.h:106
Apache Ignite API.
Definition: cache.h:48
compute::ComputeClient GetCompute()
Get client compute API.
Definition: ignite_client.h:137
Client Compute API.
Definition: compute_client.h:53
IgniteClient()
Default constructor.
Definition: ignite_client.h:56
cache::CacheClient< K, V > GetOrCreateCache(const char *name)
Get or create cache.
Definition: ignite_client.h:94
transactions::ClientTransactions ClientTransactions()
Starts transactions.
Definition: ignite_client.h:129
Cache client class template.
Definition: cache_client.h:64
Ignite thin client configuration.
Definition: ignite_client_configuration.h:39
cache::CacheClient< K, V > GetCache(const char *name)
Get cache.
Definition: ignite_client.h:82
Transactions client.
Definition: thin-client/include/ignite/thin/transactions/transactions.h:43
Ignite client class.
Definition: ignite_client.h:49