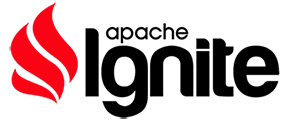 |
Apache Ignite C++
|
Go to the documentation of this file.
28 #include <ignite/common/common.h>
35 class IGNITE_IMPORT_EXPORT
Date
63 Date& operator=(
const Date& another);
70 int64_t GetMilliseconds()
const;
77 int64_t GetSeconds()
const;
134 int64_t milliseconds;
138 #endif //_IGNITE_DATE
Apache Ignite API.
Definition: cache.h:48
bool operator<(const Date &val1, const Date &val2)
Definition: date.cpp:64
bool operator>=(const Date &val1, const Date &val2)
Definition: date.cpp:79
bool operator<=(const Date &val1, const Date &val2)
Definition: date.cpp:69
Date type.
Definition: date.h:35
bool operator>(const Date &val1, const Date &val2)
Definition: date.cpp:74
bool operator!=(const Date &val1, const Date &val2)
Definition: date.cpp:59
bool operator==(const Date &val1, const Date &val2)
Definition: date.cpp:54