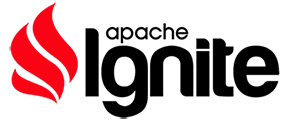 |
Apache Ignite C++
|
Go to the documentation of this file.
23 #ifndef _IGNITE_CACHE_QUERY_QUERY_SQL_FIELDS
24 #define _IGNITE_CACHE_QUERY_QUERY_SQL_FIELDS
30 #include <ignite/impl/writable_object.h>
55 distributedJoins(false),
56 enforceJoinOrder(false),
74 distributedJoins(false),
75 enforceJoinOrder(false),
90 pageSize(other.pageSize),
92 distributedJoins(other.distributedJoins),
93 enforceJoinOrder(other.enforceJoinOrder),
97 args.reserve(other.args.size());
99 typedef std::vector<impl::WritableObjectBase*>::const_iterator Iter;
101 for (Iter i = other.args.begin(); i != other.args.end(); ++i)
102 args.push_back((*i)->Copy());
127 typedef std::vector<impl::WritableObjectBase*>::const_iterator Iter;
129 for (Iter it = args.begin(); it != args.end(); ++it)
144 swap(sql, other.sql);
145 swap(schema, other.schema);
146 swap(pageSize, other.pageSize);
147 swap(loc, other.loc);
148 swap(distributedJoins, other.distributedJoins);
149 swap(enforceJoinOrder, other.enforceJoinOrder);
150 swap(lazy, other.lazy);
151 swap(args, other.args);
192 this->pageSize = pageSize;
256 return enforceJoinOrder;
271 enforceJoinOrder = enforce;
281 return distributedJoins;
294 distributedJoins = enabled;
308 args.push_back(
new impl::WritableObject<T>(arg));
319 args.push_back(
new impl::WritableObjectInt8Array(src, len));
327 std::vector<impl::WritableObjectBase*>::iterator iter;
328 for (iter = args.begin(); iter != args.end(); ++iter)
343 this->schema = schema;
370 writer.
WriteInt32(
static_cast<int32_t
>(args.size()));
372 std::vector<impl::WritableObjectBase*>::const_iterator it;
374 for (it = args.begin(); it != args.end(); ++it)
375 (*it)->Write(writer);
407 bool distributedJoins;
410 bool enforceJoinOrder;
416 std::vector<impl::WritableObjectBase*> args;
422 #endif //_IGNITE_CACHE_QUERY_QUERY_SQL_FIELDS
void SetLazy(bool lazy)
Sets lazy query execution flag.
Definition: core/include/ignite/cache/query/query_sql_fields.h:244
Apache Ignite API.
Definition: cache.h:48
SqlFieldsQuery(const SqlFieldsQuery &other)
Copy constructor.
Definition: core/include/ignite/cache/query/query_sql_fields.h:87
void WriteInt32(int32_t val)
Write 32-byte signed integer.
Definition: binary_raw_writer.cpp:72
bool IsDistributedJoins() const
Check if distributed joins are enabled for this query.
Definition: core/include/ignite/cache/query/query_sql_fields.h:279
void AddArgument(const T &arg)
Add argument.
Definition: core/include/ignite/cache/query/query_sql_fields.h:306
const std::string & GetSql() const
Get SQL string.
Definition: core/include/ignite/cache/query/query_sql_fields.h:160
SqlFieldsQuery(const std::string &sql, bool loc)
Constructor.
Definition: core/include/ignite/cache/query/query_sql_fields.h:69
void Swap(SqlFieldsQuery &other)
Efficiently swaps contents with another SqlQuery instance.
Definition: core/include/ignite/cache/query/query_sql_fields.h:138
void SetSchema(const std::string &schema)
Set schema name for the query.
Definition: core/include/ignite/cache/query/query_sql_fields.h:341
void SetPageSize(int32_t pageSize)
Set page size.
Definition: core/include/ignite/cache/query/query_sql_fields.h:190
void WriteInt32Array(const int32_t *val, int32_t len)
Write array of 32-byte signed integers.
Definition: binary_raw_writer.cpp:77
void AddInt8ArrayArgument(const int8_t *src, int32_t len)
Add array of bytes as an argument.
Definition: core/include/ignite/cache/query/query_sql_fields.h:317
void Write(binary::BinaryRawWriter &writer) const
Write query info to the stream.
Definition: core/include/ignite/cache/query/query_sql_fields.h:364
void SetLocal(bool loc)
Set local flag.
Definition: core/include/ignite/cache/query/query_sql_fields.h:212
Binary raw writer.
Definition: binary_raw_writer.h:62
SqlFieldsQuery & operator=(const SqlFieldsQuery &other)
Assignment operator.
Definition: core/include/ignite/cache/query/query_sql_fields.h:110
void SetEnforceJoinOrder(bool enforce)
Sets flag to enforce join order of tables in the query.
Definition: core/include/ignite/cache/query/query_sql_fields.h:269
void WriteString(const char *val)
Write string.
Definition: binary_raw_writer.cpp:152
~SqlFieldsQuery()
Destructor.
Definition: core/include/ignite/cache/query/query_sql_fields.h:125
const std::string & GetSchema() const
Get schema name for the query.
Definition: core/include/ignite/cache/query/query_sql_fields.h:354
void WriteBool(bool val)
Write bool.
Definition: binary_raw_writer.cpp:42
void SetSql(const std::string &sql)
Set SQL string.
Definition: core/include/ignite/cache/query/query_sql_fields.h:170
SqlFieldsQuery(const std::string &sql)
Constructor.
Definition: core/include/ignite/cache/query/query_sql_fields.h:50
void ClearArguments()
Remove all added arguments.
Definition: core/include/ignite/cache/query/query_sql_fields.h:325
Sql fields query.
Definition: core/include/ignite/cache/query/query_sql_fields.h:42
int32_t GetPageSize() const
Get page size.
Definition: core/include/ignite/cache/query/query_sql_fields.h:180
bool IsEnforceJoinOrder() const
Checks if join order of tables if enforced.
Definition: core/include/ignite/cache/query/query_sql_fields.h:254
bool IsLazy() const
Gets lazy query execution flag.
Definition: core/include/ignite/cache/query/query_sql_fields.h:224
void SetDistributedJoins(bool enabled)
Specify if distributed joins are enabled for this query.
Definition: core/include/ignite/cache/query/query_sql_fields.h:292
void WriteNull()
Write NULL value.
Definition: binary_raw_writer.cpp:177
bool IsLocal() const
Get local flag.
Definition: core/include/ignite/cache/query/query_sql_fields.h:200