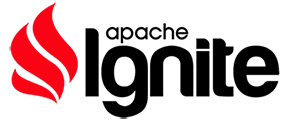 |
Apache Ignite C++
|
Go to the documentation of this file.
23 #ifndef _IGNITE_CACHE_QUERY_QUERY_SCAN
24 #define _IGNITE_CACHE_QUERY_QUERY_SCAN
56 ScanQuery(int32_t part) : part(part), pageSize(1024), loc(false)
98 this->pageSize = pageSize;
158 #endif //_IGNITE_CACHE_QUERY_QUERY_SCAN
void SetPartition(int32_t part)
Set partition to scan.
Definition: core/include/ignite/cache/query/query_scan.h:76
Apache Ignite API.
Definition: cache.h:48
void WriteInt32(int32_t val)
Write 32-byte signed integer.
Definition: binary_raw_writer.cpp:72
bool IsLocal() const
Get local flag.
Definition: core/include/ignite/cache/query/query_scan.h:106
Scan query.
Definition: core/include/ignite/cache/query/query_scan.h:40
void SetPageSize(int32_t pageSize)
Set page size.
Definition: core/include/ignite/cache/query/query_scan.h:96
Binary raw writer.
Definition: binary_raw_writer.h:62
void WriteBool(bool val)
Write bool.
Definition: binary_raw_writer.cpp:42
int32_t GetPageSize() const
Get page size.
Definition: core/include/ignite/cache/query/query_scan.h:86
ScanQuery(int32_t part)
Constructor.
Definition: core/include/ignite/cache/query/query_scan.h:56
int32_t GetPartition() const
Get partition to scan.
Definition: core/include/ignite/cache/query/query_scan.h:66
ScanQuery()
Default constructor.
Definition: core/include/ignite/cache/query/query_scan.h:46
void SetLocal(bool loc)
Set local flag.
Definition: core/include/ignite/cache/query/query_scan.h:118
void Write(binary::BinaryRawWriter &writer) const
Write query info to the stream.
Definition: core/include/ignite/cache/query/query_scan.h:128
void WriteNull()
Write NULL value.
Definition: binary_raw_writer.cpp:177