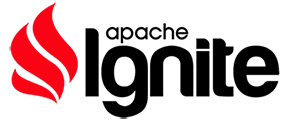 |
Apache Ignite C++
|
Go to the documentation of this file.
23 #ifndef _IGNITE_CACHE_QUERY_QUERY_FIELDS_ROW
24 #define _IGNITE_CACHE_QUERY_QUERY_FIELDS_ROW
28 #include <ignite/common/concurrent.h>
32 #include "ignite/impl/cache/query/query_fields_row_impl.h"
33 #include "ignite/impl/operations.h"
106 impl::cache::query::QueryFieldsRowImpl* impl0 = impl.Get();
109 return impl0->HasNext();
113 "Instance is not usable (did you check for error?).");
137 T res = GetNext<T>(err);
161 impl::cache::query::QueryFieldsRowImpl* impl0 = impl.Get();
164 return impl0->GetNext<T>(err);
168 "Instance is not usable (did you check for error?).");
191 impl::cache::query::QueryFieldsRowImpl* impl0 = impl.Get();
194 return impl0->GetNextInt8Array(dst, len);
198 "Instance is not usable (did you check for error?).");
215 return impl.IsValid();
220 ignite::common::concurrent::SharedPointer<impl::cache::query::QueryFieldsRowImpl> impl;
226 #endif //_IGNITE_CACHE_QUERY_QUERY_FIELDS_ROW
int32_t GetNextInt8Array(int8_t *dst, int32_t len)
Get next entry assuming it's an array of 8-byte signed integers.
Definition: core/include/ignite/cache/query/query_fields_row.h:189
QueryFieldsRow()
Default constructor.
Definition: core/include/ignite/cache/query/query_fields_row.h:57
Apache Ignite API.
Definition: cache.h:48
T GetNext(IgniteError &err)
Get next entry.
Definition: core/include/ignite/cache/query/query_fields_row.h:159
bool HasNext(IgniteError &err)
Check whether next entry exists.
Definition: core/include/ignite/cache/query/query_fields_row.h:104
QueryFieldsRow(impl::cache::query::QueryFieldsRowImpl *impl)
Constructor.
Definition: core/include/ignite/cache/query/query_fields_row.h:69
T GetNext()
Get next entry.
Definition: core/include/ignite/cache/query/query_fields_row.h:133
bool IsValid() const
Check if the instance is valid.
Definition: core/include/ignite/cache/query/query_fields_row.h:213
static const int IGNITE_ERR_GENERIC
Generic Ignite error.
Definition: ignite_error.h:131
bool HasNext()
Check whether next entry exists.
Definition: core/include/ignite/cache/query/query_fields_row.h:83
static void ThrowIfNeeded(const IgniteError &err)
Throw an error if code is not IGNITE_SUCCESS.
Definition: ignite_error.cpp:27
Query fields cursor.
Definition: core/include/ignite/cache/query/query_fields_row.h:49
Ignite error information.
Definition: ignite_error.h:94