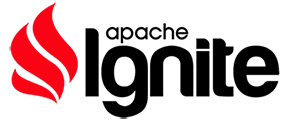 |
Apache Ignite C++
|
Go to the documentation of this file.
23 #ifndef _IGNITE_CACHE_QUERY_QUERY_CURSOR
24 #define _IGNITE_CACHE_QUERY_QUERY_CURSOR
28 #include <ignite/common/concurrent.h>
32 #include "ignite/impl/cache/query/query_impl.h"
33 #include "ignite/impl/operations.h"
53 template<
typename K,
typename V>
75 QueryCursor(impl::cache::query::QueryCursorImpl* impl) : impl(impl)
112 impl::cache::query::QueryCursorImpl* impl0 = impl.Get();
115 return impl0->HasNext(err);
119 "Instance is not usable (did you check for error?).");
158 impl::cache::query::QueryCursorImpl* impl0 = impl.Get();
164 impl::Out2Operation<K, V> outOp(key, val);
166 impl0->GetNext(outOp, err);
173 "Instance is not usable (did you check for error?).");
208 impl::cache::query::QueryCursorImpl* impl0 = impl.Get();
211 impl::OutQueryGetAllOperation<K, V> outOp(res);
213 impl0->GetAll(outOp, err);
217 "Instance is not usable (did you check for error?).");
227 template<
typename OutIter>
230 impl::cache::query::QueryCursorImpl* impl0 = impl.Get();
233 impl::OutQueryGetAllOperationIter<K, V, OutIter> outOp(iter);
235 impl0->GetAll(outOp);
240 "Instance is not usable (did you check for error?).");
257 return impl.IsValid();
262 ignite::common::concurrent::SharedPointer<impl::cache::query::QueryCursorImpl> impl;
268 #endif //_IGNITE_CACHE_QUERY_QUERY_CURSOR
Apache Ignite API.
Definition: cache.h:48
void GetAll(std::vector< CacheEntry< K, V > > &res)
Get all entries.
Definition: core/include/ignite/cache/query/query_cursor.h:188
bool HasNext()
Check whether next entry exists.
Definition: core/include/ignite/cache/query/query_cursor.h:89
Cache entry class template.
Definition: core/include/ignite/cache/cache_entry.h:40
Query cursor class template.
Definition: core/include/ignite/cache/query/query_cursor.h:54
QueryCursor(impl::cache::query::QueryCursorImpl *impl)
Constructor.
Definition: core/include/ignite/cache/query/query_cursor.h:75
CacheEntry< K, V > GetNext()
Get next entry.
Definition: core/include/ignite/cache/query/query_cursor.h:134
static const int IGNITE_ERR_GENERIC
Generic Ignite error.
Definition: ignite_error.h:131
void GetAll(OutIter iter)
Get all entries.
Definition: core/include/ignite/cache/query/query_cursor.h:228
bool IsValid() const
Check if the instance is valid.
Definition: core/include/ignite/cache/query/query_cursor.h:255
CacheEntry< K, V > GetNext(IgniteError &err)
Get next entry.
Definition: core/include/ignite/cache/query/query_cursor.h:156
void GetAll(std::vector< CacheEntry< K, V > > &res, IgniteError &err)
Get all entries.
Definition: core/include/ignite/cache/query/query_cursor.h:206
static void ThrowIfNeeded(const IgniteError &err)
Throw an error if code is not IGNITE_SUCCESS.
Definition: ignite_error.cpp:27
QueryCursor()
Default constructor.
Definition: core/include/ignite/cache/query/query_cursor.h:63
bool HasNext(IgniteError &err)
Check whether next entry exists.
Definition: core/include/ignite/cache/query/query_cursor.h:110
Ignite error information.
Definition: ignite_error.h:94