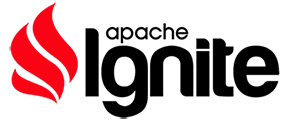 |
Apache Ignite C++
|
Go to the documentation of this file.
23 #ifndef _IGNITE_CACHE_EVENT_CACHE_ENTRY_EVENT
24 #define _IGNITE_CACHE_EVENT_CACHE_ENTRY_EVENT
53 static T FromInt8(int8_t val)
61 return static_cast<T>(val);
66 "Unsupported CacheEntryEventType",
"val", val);
78 template<
typename K,
typename V>
103 oldVal(other.oldVal),
104 hasOldValue(other.hasOldValue),
105 eventType(other.eventType)
130 oldVal = other.oldVal;
131 hasOldValue = other.hasOldValue;
132 eventType = other.eventType;
182 int8_t eventTypeByte = reader.
ReadInt8();
183 this->eventType = CacheEntryEventType::FromInt8(eventTypeByte);
199 #endif //_IGNITE_CACHE_EVENT_CACHE_ENTRY_EVENT
@ REMOVE
An event type indicating that the cache entry was removed.
Definition: core/include/ignite/cache/event/cache_entry_event.h:47
Binary raw reader.
Definition: binary_raw_reader.h:57
Apache Ignite API.
Definition: cache.h:48
@ CREATE
An event type indicating that the cache entry was created.
Definition: core/include/ignite/cache/event/cache_entry_event.h:41
Cache entry event type.
Definition: core/include/ignite/cache/event/cache_entry_event.h:36
CacheEntryEvent & operator=(const CacheEntryEvent< K, V > &other)
Assignment operator.
Definition: core/include/ignite/cache/event/cache_entry_event.h:124
CacheEntryEventType::T GetEventType() const
Get event type.
Definition: core/include/ignite/cache/event/cache_entry_event.h:165
Cache entry class template.
Definition: core/include/ignite/cache/cache_entry.h:40
K key
Key.
Definition: core/include/ignite/cache/cache_entry.h:154
@ EXPIRE
An event type indicating that the cache entry was removed by expiration policy.
Definition: core/include/ignite/cache/event/cache_entry_event.h:50
bool TryReadObject(T &res)
Try read object.
Definition: binary_raw_reader.h:486
T ReadObject()
Read object.
Definition: binary_raw_reader.h:458
Cache entry event class template.
Definition: core/include/ignite/cache/event/cache_entry_event.h:79
CacheEntryEvent()
Default constructor.
Definition: core/include/ignite/cache/event/cache_entry_event.h:87
const V & GetOldValue() const
Get old value.
Definition: core/include/ignite/cache/event/cache_entry_event.h:143
CacheEntry & operator=(const CacheEntry &other)
Assignment operator.
Definition: core/include/ignite/cache/cache_entry.h:110
T
Definition: core/include/ignite/cache/event/cache_entry_event.h:38
CacheEntryEvent(const CacheEntryEvent< K, V > &other)
Copy constructor.
Definition: core/include/ignite/cache/event/cache_entry_event.h:101
V val
Value.
Definition: core/include/ignite/cache/cache_entry.h:157
void Read(binary::BinaryRawReader &reader)
Reads cache event using provided raw reader.
Definition: core/include/ignite/cache/event/cache_entry_event.h:175
bool hasValue
Indicates whether value exists.
Definition: core/include/ignite/cache/cache_entry.h:160
virtual ~CacheEntryEvent()
Destructor.
Definition: core/include/ignite/cache/event/cache_entry_event.h:113
int8_t ReadInt8()
Read 8-byte signed integer.
Definition: binary_raw_reader.cpp:32
static const int IGNITE_ERR_BINARY
Binary error.
Definition: ignite_error.h:125
bool HasOldValue() const
Check if the old value exists.
Definition: core/include/ignite/cache/event/cache_entry_event.h:153
@ UPDATE
An event type indicating that the cache entry was updated.
Definition: core/include/ignite/cache/event/cache_entry_event.h:44