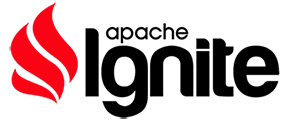 |
Apache Ignite C++
|
Go to the documentation of this file.
23 #ifndef _IGNITE_THIN_CACHE_QUERY_CONTINUOUS_CONTINUOUS_QUERY_CLIENT
24 #define _IGNITE_THIN_CACHE_QUERY_CONTINUOUS_CONTINUOUS_QUERY_CLIENT
53 template<
typename K,
typename V>
60 enum { DEFAULT_BUFFER_SIZE = 1 };
65 enum { DEFAULT_TIME_INTERVAL = 0 };
82 bufferSize(DEFAULT_BUFFER_SIZE),
83 timeInterval(DEFAULT_TIME_INTERVAL),
84 includeExpired(false),
165 includeExpired = val;
180 return includeExpired;
202 return *listener.Get();
212 return *listener.Get();
251 int64_t timeInterval;
268 #endif //_IGNITE_THIN_CACHE_QUERY_CONTINUOUS_CONTINUOUS_QUERY_CLIENT
Apache Ignite API.
Definition: cache.h:48
int64_t GetTimeInterval() const
Get time interval.
Definition: continuous_query_client.h:148
void SetIncludeExpired(bool val)
Sets a value indicating whether to notify about Expired events.
Definition: continuous_query_client.h:163
int32_t GetBufferSize() const
Get buffer size.
Definition: continuous_query_client.h:114
Cache entry event listener.
Definition: thin-client/include/ignite/thin/cache/event/cache_entry_event_listener.h:42
Continuous query client.
Definition: continuous_query_client.h:54
void SetTimeInterval(int64_t val)
Set time interval.
Definition: continuous_query_client.h:131
const event::JavaCacheEntryEventFilter & GetJavaFilter() const
Get remote Java filter reference.
Definition: continuous_query_client.h:241
ContinuousQueryClient(Reference< event::CacheEntryEventListener< K, V > > lsnr)
Constructor.
Definition: continuous_query_client.h:81
void SetJavaFilter(const event::JavaCacheEntryEventFilter &fltr)
Set Java event filter to be used on server to determine what events should be transferred to local ev...
Definition: continuous_query_client.h:221
~ContinuousQueryClient()
Destructor.
Definition: continuous_query_client.h:70
event::CacheEntryEventListener< K, V > & GetListener()
Get cache entry event listener.
Definition: continuous_query_client.h:210
void SetBufferSize(int32_t val)
Set buffer size.
Definition: continuous_query_client.h:100
const event::CacheEntryEventListener< K, V > & GetListener() const
Get cache entry event listener.
Definition: continuous_query_client.h:200
void SetListener(Reference< event::CacheEntryEventListener< K, V > > lsnr)
Set cache entry event listener.
Definition: continuous_query_client.h:190
bool GetIncludeExpired() const
Gets a value indicating whether to notify about Expired events.
Definition: continuous_query_client.h:178
event::JavaCacheEntryEventFilter & GetJavaFilter()
Get remote Java filter reference.
Definition: continuous_query_client.h:231
Reference class.
Definition: reference.h:35
Java cache entry event filter.
Definition: thin-client/include/ignite/thin/cache/event/java_cache_entry_event_filter.h:55