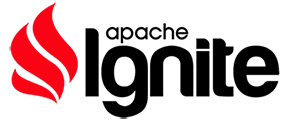 |
Apache Ignite C++
|
Go to the documentation of this file.
23 #ifndef _IGNITE_COMPUTE_COMPUTE
24 #define _IGNITE_COMPUTE_COMPUTE
26 #include <ignite/common/common.h>
32 #include <ignite/impl/compute/compute_impl.h>
84 Compute(common::concurrent::SharedPointer<impl::compute::ComputeImpl> impl) :
106 template<
typename R,
typename K,
typename F>
107 R
AffinityCall(
const std::string& cacheName,
const K& key,
const F& func)
109 return impl.Get()->AffinityCallAsync<R, K, F>(cacheName, key, func).GetValue();
129 template<
typename R,
typename K,
typename F>
132 return impl.Get()->AffinityCallAsync<R, K, F>(cacheName, key, func);
147 template<
typename K,
typename F>
148 void AffinityRun(
const std::string& cacheName,
const K& key,
const F& action)
150 return impl.Get()->AffinityRunAsync<K, F>(cacheName, key, action).GetValue();
167 template<
typename K,
typename F>
170 return impl.Get()->AffinityRunAsync<K, F>(cacheName, key, action);
186 template<
typename R,
typename F>
189 return impl.Get()->CallAsync<R, F>(func).GetValue();
206 template<
typename R,
typename F>
209 return impl.Get()->CallAsync<R, F>(func);
224 return impl.Get()->RunAsync<F>(action).GetValue();
240 return impl.Get()->RunAsync<F>(action);
254 template<
typename R,
typename F>
257 return impl.Get()->BroadcastAsync<R, F>(func).GetValue();
271 impl.Get()->BroadcastAsync<F,
false>(func).GetValue();
287 template<
typename R,
typename F>
290 return impl.Get()->BroadcastAsync<R, F>(func);
306 return impl.Get()->BroadcastAsync<F,
false>(func);
320 template<
typename R,
typename A>
323 return impl.Get()->ExecuteJavaTask<R, A>(taskName, taskArg);
338 return impl.Get()->ExecuteJavaTask<R>(taskName);
352 template<
typename R,
typename A>
355 return impl.Get()->ExecuteJavaTaskAsync<R, A>(taskName, taskArg);
370 return impl.Get()->ExecuteJavaTaskAsync<R>(taskName);
375 common::concurrent::SharedPointer<impl::compute::ComputeImpl> impl;
380 #endif //_IGNITE_COMPUTE_COMPUTE
Defines compute grid functionality for executing tasks and closures over nodes in the ClusterGroup.
Definition: compute.h:74
Apache Ignite API.
Definition: cache.h:48
std::vector< R > Broadcast(const F &func)
Broadcasts provided ComputeFunc to all nodes in the cluster group.
Definition: compute.h:255
Future< void > RunAsync(const F &action)
Asyncronuously runs provided ComputeFunc on a node within the underlying cluster group.
Definition: compute.h:238
Future< R > CallAsync(const F &func)
Asyncronuously calls provided ComputeFunc on a node within the underlying cluster group.
Definition: compute.h:207
Future class template.
Definition: future.h:46
Future< R > ExecuteJavaTaskAsync(const std::string &taskName)
Asynchronously executes given Java task on the grid projection.
Definition: compute.h:368
Future< std::vector< R > > BroadcastAsync(const F &func)
Asyncronuously broadcasts provided ComputeFunc to all nodes in the cluster group.
Definition: compute.h:288
void Broadcast(const F &func)
Broadcasts provided ComputeFunc to all nodes in the cluster group.
Definition: compute.h:269
Specialization for void type.
Definition: future.h:167
void Run(const F &action)
Runs provided ComputeFunc on a node within the underlying cluster group.
Definition: compute.h:222
Future< void > AffinityRunAsync(const std::string &cacheName, const K &key, const F &action)
Executes given job asynchronously on the node where data for provided affinity key is located (a....
Definition: compute.h:168
Compute(common::concurrent::SharedPointer< impl::compute::ComputeImpl > impl)
Constructor.
Definition: compute.h:84
Future< R > ExecuteJavaTaskAsync(const std::string &taskName, const A &taskArg)
Asynchronously executes given Java task on the grid projection.
Definition: compute.h:353
Future< R > AffinityCallAsync(const std::string &cacheName, const K &key, const F &func)
Executes given job asynchronously on the node where data for provided affinity key is located (a....
Definition: compute.h:130
R AffinityCall(const std::string &cacheName, const K &key, const F &func)
Executes given job on the node where data for provided affinity key is located (a....
Definition: compute.h:107
R ExecuteJavaTask(const std::string &taskName, const A &taskArg)
Executes given Java task on the grid projection.
Definition: compute.h:321
R ExecuteJavaTask(const std::string &taskName)
Executes given Java task on the grid projection.
Definition: compute.h:336
Future< void > BroadcastAsync(const F &func)
Asyncronuously broadcasts provided ComputeFunc to all nodes in the cluster group.
Definition: compute.h:304
void AffinityRun(const std::string &cacheName, const K &key, const F &action)
Executes given job on the node where data for provided affinity key is located (a....
Definition: compute.h:148
R Call(const F &func)
Calls provided ComputeFunc on a node within the underlying cluster group.
Definition: compute.h:187