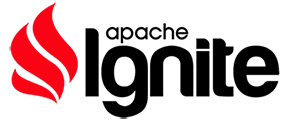 |
Apache Ignite C++
|
Go to the documentation of this file.
23 #ifndef _IGNITE_THIN_CACHE_CACHE_CLIENT
24 #define _IGNITE_THIN_CACHE_CACHE_CLIENT
26 #include <ignite/common/concurrent.h>
35 #include <ignite/impl/thin/writable.h>
36 #include <ignite/impl/thin/writable_key.h>
38 #include <ignite/impl/thin/readable.h>
39 #include <ignite/impl/thin/cache/cache_client_proxy.h>
40 #include <ignite/impl/thin/cache/continuous/continuous_query_client_holder.h>
63 template<
typename K,
typename V>
66 friend class impl::thin::cache::CacheClientProxy;
80 explicit CacheClient(
const common::concurrent::SharedPointer<void>& impl) :
110 impl::thin::WritableKeyImpl<KeyType> wrKey(key);
111 impl::thin::WritableImpl<ValueType> wrValue(value);
113 proxy.Put(wrKey, wrValue);
123 template<
typename InIter>
126 impl::thin::WritableMapImpl<K, V, InIter> wrSeq(begin, end);
137 template<
typename Map>
140 PutAll(vals.begin(), vals.end());
151 impl::thin::WritableKeyImpl<KeyType> wrKey(key);
154 proxy.Get(wrKey, rdValue);
182 template<
typename InIter,
typename OutIter>
183 void GetAll(InIter begin, InIter end, OutIter dst)
185 impl::thin::WritableSetImpl<K, InIter> wrSeq(begin, end);
186 impl::thin::ReadableContainerImpl< std::pair<K, V>, OutIter> rdSeq(dst);
188 proxy.GetAll(wrSeq, rdSeq);
200 template<
typename Set,
typename Map>
203 return GetAll(keys.begin(), keys.end(), std::inserter(res, res.end()));
220 impl::thin::WritableKeyImpl<KeyType> wrKey(key);
221 impl::thin::WritableImpl<ValueType> wrValue(value);
223 return proxy.Replace(wrKey, wrValue);
237 impl::thin::WritableKeyImpl<KeyType> wrKey(key);
238 impl::thin::WritableImpl<ValueType> wrOldVal(oldVal);
239 impl::thin::WritableImpl<ValueType> wrNewVal(newVal);
241 return proxy.Replace(wrKey, wrOldVal, wrNewVal);
252 impl::thin::WritableKeyImpl<KeyType> wrKey(key);
254 return proxy.ContainsKey(wrKey);
263 template<
typename Set>
276 template<
typename InIter>
279 impl::thin::WritableSetImpl<K, InIter> wrSeq(begin, end);
281 return proxy.ContainsKeys(wrSeq);
295 return proxy.GetSize(peekModes);
312 impl::thin::WritableKeyImpl<KeyType> wrKey(key);
314 return proxy.Remove(wrKey);
327 impl::thin::WritableKeyImpl<KeyType> wrKey(key);
328 impl::thin::WritableImpl<ValueType> wrVal(val);
330 return proxy.Remove(wrKey, wrVal);
339 template<
typename Set>
352 template<
typename InIter>
355 impl::thin::WritableSetImpl<K, InIter> wrSeq(begin, end);
357 proxy.RemoveAll(wrSeq);
378 impl::thin::WritableKeyImpl<KeyType> wrKey(key);
397 template<
typename Set>
410 template<
typename InIter>
413 impl::thin::WritableSetImpl<K, InIter> wrSeq(begin, end);
415 proxy.ClearAll(wrSeq);
429 impl::thin::WritableKeyImpl<KeyType> wrKey(key);
430 impl::thin::WritableImpl<ValueType> wrValIn(valIn);
433 proxy.GetAndPut(wrKey, wrValIn, rdValOut);
463 impl::thin::WritableKeyImpl<KeyType> wrKey(key);
466 proxy.GetAndRemove(wrKey, rdValOut);
496 impl::thin::WritableKeyImpl<KeyType> wrKey(key);
497 impl::thin::WritableImpl<ValueType> wrValIn(valIn);
500 proxy.GetAndReplace(wrKey, wrValIn, rdValOut);
531 impl::thin::WritableKeyImpl<KeyType> wrKey(key);
532 impl::thin::WritableImpl<ValueType> wrValIn(val);
534 return proxy.PutIfAbsent(wrKey, wrValIn);
558 impl::thin::WritableKeyImpl<KeyType> wrKey(key);
559 impl::thin::WritableImpl<ValueType> wrValIn(valIn);
562 proxy.GetAndPutIfAbsent(wrKey, wrValIn, rdValOut);
601 return proxy.Query(qry);
624 using namespace impl::thin::cache::query::continuous;
626 SP_ContinuousQueryClientHolderBase holder(
new ContinuousQueryClientHolder<K, V>(continuousQuery));
628 return proxy.QueryContinuous(holder, continuousQuery.
GetJavaFilter());
649 impl::thin::cache::CacheClientProxy proxy;
655 #endif // _IGNITE_THIN_CACHE_CACHE_CLIENT
void PutAll(InIter begin, InIter end)
Stores given key-value pairs in cache.
Definition: cache_client.h:124
Apache Ignite API.
Definition: cache.h:48
void RemoveAll()
Removes all mappings from cache.
Definition: cache_client.h:365
ValueType GetAndRemove(const KeyType &key)
Atomically removes the entry for a key only if currently mapped to some value.
Definition: cache_client.h:476
~CacheClient()
Destructor.
Definition: cache_client.h:97
bool Remove(const KeyType &key)
Removes given key mapping from cache.
Definition: cache_client.h:310
Continuous query client.
Definition: continuous_query_client.h:54
void RemoveAll(InIter begin, InIter end)
Removes given key mappings from cache.
Definition: cache_client.h:353
ValueType GetAndReplace(const KeyType &key, const ValueType &valIn)
Atomically replaces the value for a given key if and only if there is a value currently mapped by the...
Definition: cache_client.h:512
Query fields cursor.
Definition: thin-client/include/ignite/thin/cache/query/query_fields_cursor.h:48
bool ContainsKeys(const Set &keys)
Check if cache contains mapping for these keys.
Definition: cache_client.h:264
int64_t GetSize(int32_t peekModes)
Gets the number of all entries cached across all nodes.
Definition: cache_client.h:293
Continuous query handle client.
Definition: thin-client/include/ignite/thin/cache/query/continuous/continuous_query_handle.h:43
SQL fields query for thin client.
Definition: thin-client/include/ignite/thin/cache/query/query_sql_fields.h:52
CacheClient(const common::concurrent::SharedPointer< void > &impl)
Constructor.
Definition: cache_client.h:80
ValueType GetAndPutIfAbsent(const KeyType &key, const ValueType &valIn)
Stores given key-value pair in cache only if cache had no previous mapping for it.
Definition: cache_client.h:584
void RemoveAll(const Set &keys)
Removes given key mappings from cache.
Definition: cache_client.h:340
bool ContainsKey(const KeyType &key)
Check if the cache contains a value for the specified key.
Definition: cache_client.h:250
query::continuous::ContinuousQueryHandleClient QueryContinuous(query::continuous::ContinuousQueryClient< K, V > continuousQuery)
Starts the continuous query execution.
Definition: cache_client.h:621
ValueType GetAndPut(const KeyType &key, const ValueType &valIn)
Associates the specified value with the specified key in this cache, returning an existing value if o...
Definition: cache_client.h:445
K KeyType
Key type.
Definition: cache_client.h:70
Cache client class template.
Definition: cache_client.h:64
query::QueryFieldsCursor Query(const query::SqlFieldsQuery &qry)
Perform SQL fields query.
Definition: cache_client.h:599
V ValueType
Value type.
Definition: cache_client.h:73
bool Replace(const KeyType &key, const ValueType &oldVal, const ValueType &newVal)
Stores given key-value pair in cache only if the previous value is equal to the old value passed as a...
Definition: cache_client.h:235
bool PutIfAbsent(const KeyType &key, const ValueType &val)
Atomically associates the specified key with the given value if it is not already associated with a v...
Definition: cache_client.h:529
void Get(const KeyType &key, ValueType &value)
Get value from the cache.
Definition: cache_client.h:149
CacheClient()
Default constructor.
Definition: cache_client.h:89
query::QueryCursor< KeyType, ValueType > Query(const query::ScanQuery &qry)
Perform scan query.
Definition: cache_client.h:610
void GetAndPut(const KeyType &key, const ValueType &valIn, ValueType &valOut)
Associates the specified value with the specified key in this cache, returning an existing value if o...
Definition: cache_client.h:427
void ClearAll(InIter begin, InIter end)
Clear entries from the cache and swap storage, without notifying listeners or CacheWriters.
Definition: cache_client.h:411
void Put(const KeyType &key, const ValueType &value)
Associate the specified value with the specified key in the cache.
Definition: cache_client.h:108
Scan query.
Definition: thin-client/include/ignite/thin/cache/query/query_scan.h:52
Query cursor class template.
Definition: thin-client/include/ignite/thin/cache/query/query_cursor.h:57
void GetAll(InIter begin, InIter end, OutIter dst)
Retrieves values mapped to the specified keys from cache.
Definition: cache_client.h:183
void GetAll(const Set &keys, Map &res)
Retrieves values mapped to the specified keys from cache.
Definition: cache_client.h:201
bool Replace(const K &key, const V &value)
Stores given key-value pair in cache only if there is a previous mapping for it.
Definition: cache_client.h:218
void Clear(const KeyType &key)
Clear entry from the cache and swap storage, without notifying listeners or CacheWriters.
Definition: cache_client.h:376
void PutAll(const Map &vals)
Stores given key-value pairs in cache.
Definition: cache_client.h:138
void GetAndRemove(const KeyType &key, ValueType &valOut)
Atomically removes the entry for a key only if currently mapped to some value.
Definition: cache_client.h:461
event::JavaCacheEntryEventFilter & GetJavaFilter()
Get remote Java filter reference.
Definition: continuous_query_client.h:231
void Clear()
Clear cache.
Definition: cache_client.h:386
void GetAndReplace(const KeyType &key, const ValueType &valIn, ValueType &valOut)
Atomically replaces the value for a given key if and only if there is a value currently mapped by the...
Definition: cache_client.h:494
void GetAndPutIfAbsent(const KeyType &key, const ValueType &valIn, ValueType &valOut)
Stores given key-value pair in cache only if cache had no previous mapping for it.
Definition: cache_client.h:556
Definition: thin-client/include/ignite/thin/cache/cache_entry.h:36
void RefreshAffinityMapping()
Refresh affinity mapping.
Definition: cache_client.h:642
bool ContainsKeys(InIter begin, InIter end)
Check if cache contains mapping for these keys.
Definition: cache_client.h:277
bool Remove(const KeyType &key, const ValueType &val)
Removes given key mapping from cache if one exists and value is equal to the passed in value.
Definition: cache_client.h:325
void ClearAll(const Set &keys)
Clear entries from the cache and swap storage, without notifying listeners or CacheWriters.
Definition: cache_client.h:398
ValueType Get(const KeyType &key)
Get value from cache.
Definition: cache_client.h:163