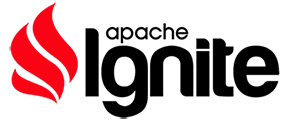 |
Apache Ignite C++
|
Go to the documentation of this file.
23 #ifndef _IGNITE_CACHE_AFFINITY
24 #define _IGNITE_CACHE_AFFINITY
28 #include <ignite/impl/cache/cache_affinity_impl.h>
63 return impl.Get()->GetPartitions();
74 return impl.Get()->GetPartition(key);
86 return impl.Get()->IsPrimary(node, key);
98 return impl.Get()->IsBackup(node, key);
113 return impl.Get()->IsPrimaryOrBackup(node, key);
124 return impl.Get()->GetPrimaryPartitions(node);
135 return impl.Get()->GetBackupPartitions(node);
146 return impl.Get()->GetAllPartitions(node);
157 template <
typename TR>
160 return impl.Get()->template GetAffinityKey<K, TR>(key);
171 std::map<cluster::ClusterNode, std::vector<K> >
MapKeysToNodes(
const std::vector<K>& keys)
173 return impl.Get()->MapKeysToNodes(keys);
186 return impl.Get()->MapKeyToNode(key);
198 return impl.Get()->MapKeyToPrimaryAndBackups(key);
209 return impl.Get()->MapPartitionToNode(part);
220 return impl.Get()->MapPartitionsToNodes(parts);
232 return impl.Get()->MapPartitionToPrimaryAndBackups(part);
236 impl::cache::SP_CacheAffinityImpl impl;
241 #endif //_IGNITE_CACHE_AFFINITY
bool IsBackup(cluster::ClusterNode node, const K &key)
Return true if local node is one of the backup nodes for given key.
Definition: cache_affinity.h:96
Apache Ignite API.
Definition: cache.h:48
std::vector< int32_t > GetAllPartitions(cluster::ClusterNode node)
Get partition ids for which given cluster node has any ownership (either primary or backup).
Definition: cache_affinity.h:144
bool IsPrimary(cluster::ClusterNode node, const K &key)
Return true if given node is the primary node for given key.
Definition: cache_affinity.h:84
std::vector< int32_t > GetPrimaryPartitions(cluster::ClusterNode node)
Get partition ids for which the given cluster node has primary ownership.
Definition: cache_affinity.h:122
std::vector< cluster::ClusterNode > MapKeyToPrimaryAndBackups(const K &key)
Get primary and backup nodes for the key.
Definition: cache_affinity.h:196
int32_t GetPartition(const K &key)
Get partition id for the given key.
Definition: cache_affinity.h:72
std::vector< int32_t > GetBackupPartitions(cluster::ClusterNode node)
Get partition ids for which given cluster node has backup ownership.
Definition: cache_affinity.h:133
std::vector< cluster::ClusterNode > MapPartitionToPrimaryAndBackups(int32_t part)
Get primary and backup nodes for partition.
Definition: cache_affinity.h:230
int32_t GetPartitions()
Get number of partitions in cache according to configured affinity function.
Definition: cache_affinity.h:61
std::map< cluster::ClusterNode, std::vector< K > > MapKeysToNodes(const std::vector< K > &keys)
This method provides ability to detect which keys are mapped to which nodes.
Definition: cache_affinity.h:171
Provides affinity information to detect which node is primary and which nodes are backups for a parti...
Definition: cache_affinity.h:42
cluster::ClusterNode MapPartitionToNode(int32_t part)
Get primary node for the given partition.
Definition: cache_affinity.h:207
TR GetAffinityKey(const K &key)
Map passed in key to a key which will be used for node affinity.
Definition: cache_affinity.h:158
bool IsPrimaryOrBackup(cluster::ClusterNode node, const K &key)
Returns true if local node is primary or one of the backup nodes.
Definition: cache_affinity.h:111
CacheAffinity(impl::cache::SP_CacheAffinityImpl impl)
Constructor.
Definition: cache_affinity.h:50
std::map< int32_t, cluster::ClusterNode > MapPartitionsToNodes(const std::vector< int32_t > &parts)
Get primary nodes for the given partitions.
Definition: cache_affinity.h:218
Interface representing a single cluster node.
Definition: cluster_node.h:36
cluster::ClusterNode MapKeyToNode(const K &key)
This method provides ability to detect to which primary node the given key is mapped.
Definition: cache_affinity.h:184