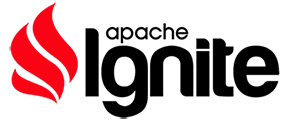 |
Apache Ignite C++
|
Go to the documentation of this file.
23 #ifndef _IGNITE_CACHE_CACHE
24 #define _IGNITE_CACHE_CACHE
29 #include <ignite/common/common.h>
30 #include <ignite/common/concurrent.h>
42 #include <ignite/impl/cache/cache_impl.h>
43 #include <ignite/impl/cache/cache_entry_processor_holder.h>
44 #include <ignite/impl/operations.h>
45 #include <ignite/impl/module_manager.h>
67 template<
typename K,
typename V>
68 class IGNITE_IMPORT_EXPORT
Cache
78 Cache(impl::cache::CacheImpl* impl) :
93 return impl.Get()->GetName();
108 bool res = IsEmpty(err);
126 return Size(err) == 0;
141 bool res = ContainsKey(key, err);
159 impl::In1Operation<K> op(key);
161 return impl.Get()->ContainsKey(op, err);
176 bool res = ContainsKeys(keys, err);
192 template<
typename InputIter>
197 impl::InIterOperation<K, V, InputIter> op(begin, end);
199 bool res = impl.Get()->ContainsKeys(op, err);
217 impl::InSetOperation<K> op(keys);
219 return impl.Get()->ContainsKeys(op, err);
239 V res = LocalPeek(key, peekModes, err);
264 impl::InCacheLocalPeekOperation<K> inOp(key, peekModes);
265 impl::Out1Operation<V> outOp(val);
267 impl.Get()->LocalPeek(inOp, outOp, err);
288 V res = Get(key, err);
311 impl::In1Operation<K> inOp(key);
312 impl::Out1Operation<V> outOp(val);
314 impl.Get()->Get(inOp, outOp, err);
331 std::map<K, V>
GetAll(
const std::set<K>& keys)
335 std::map<K, V> res = GetAll(keys, err);
359 impl::InSetOperation<K> inOp(keys);
360 impl::OutMapOperation<K, V> outOp(res);
362 impl.Get()->GetAll(inOp, outOp, err);
380 template<
typename InIter,
typename OutIter>
381 void GetAll(InIter begin, InIter end, OutIter dst)
385 impl::InIterOperation<K, V, InIter> inOp(begin, end);
386 impl::OutMapIterOperation<K, V, OutIter> outOp(dst);
388 impl.Get()->GetAll(inOp, outOp, err);
403 void Put(
const K& key,
const V& val)
425 impl::In2Operation<K, V> op(key, val);
427 impl.Get()->Put(op, err);
460 impl::InMapOperation<K, V> op(vals);
462 impl.Get()->PutAll(op, err);
478 template<
typename Iter>
483 impl::InIterOperation<K, V, Iter> op(begin, end);
485 impl.Get()->PutAll(op, err);
505 V res = GetAndPut(key, val, err);
528 impl::In2Operation<K, V> inOp(key, val);
529 impl::Out1Operation<V> outOp(oldVal);
531 impl.Get()->GetAndPut(inOp, outOp, err);
551 V res = GetAndReplace(key, val, err);
574 impl::In2Operation<K, V> inOp(key, val);
575 impl::Out1Operation<V> outOp(oldVal);
577 impl.Get()->GetAndReplace(inOp, outOp, err);
594 V res = GetAndRemove(key, err);
614 impl::In1Operation<K> inOp(key);
615 impl::Out1Operation<V> outOp(oldVal);
617 impl.Get()->GetAndRemove(inOp, outOp, err);
636 bool res = PutIfAbsent(key, val, err);
656 impl::In2Operation<K, V> op(key, val);
658 return impl.Get()->PutIfAbsent(op, err);
683 V res = GetAndPutIfAbsent(key, val, err);
713 impl::In2Operation<K, V> inOp(key, val);
714 impl::Out1Operation<V> outOp(oldVal);
716 impl.Get()->GetAndPutIfAbsent(inOp, outOp, err);
740 bool res = Replace(key, val, err);
765 impl::In2Operation<K, V> op(key, val);
767 return impl.Get()->Replace(op, err);
782 bool Replace(
const K& key,
const V& oldVal,
const V& newVal)
786 bool res = Replace(key, oldVal, newVal, err);
808 impl::In3Operation<K, V, V> op(key, oldVal, newVal);
810 return impl.Get()->ReplaceIfEqual(op, err);
827 LocalEvict(keys, err);
845 impl::InSetOperation<K> op(keys);
847 impl.Get()->LocalEvict(op, err);
861 template<
typename Iter>
866 impl::InIterOperation<K, V, Iter> op(begin, end);
868 impl.Get()->LocalEvict(op, err);
896 impl.Get()->Clear(err);
927 impl::In1Operation<K> op(key);
929 impl.Get()->Clear(op, err);
960 impl::InSetOperation<K> op(keys);
962 impl.Get()->ClearAll(op, err);
974 template<
typename Iter>
979 impl::InIterOperation<K, V, Iter> op(begin, end);
981 impl.Get()->ClearAll(op, err);
1001 LocalClear(key, err);
1020 impl::In1Operation<K> op(key);
1022 impl.Get()->LocalClear(op, err);
1040 LocalClearAll(keys, err);
1059 impl::InSetOperation<K> op(keys);
1061 impl.Get()->LocalClearAll(op, err);
1076 template<
typename Iter>
1081 impl::InIterOperation<K, V, Iter> op(begin, end);
1083 impl.Get()->LocalClearAll(op, err);
1107 bool res = Remove(key, err);
1132 impl::In1Operation<K> op(key);
1134 return impl.Get()->Remove(op, err);
1152 bool res = Remove(key, val, err);
1173 impl::In2Operation<K, V> op(key, val);
1175 return impl.Get()->RemoveIfEqual(op, err);
1191 RemoveAll(keys, err);
1208 impl::InSetOperation<K> op(keys);
1210 impl.Get()->RemoveAll(op, err);
1226 template<
typename Iter>
1231 impl::InIterOperation<K, V, Iter> op(begin, end);
1233 impl.Get()->RemoveAll(op, err);
1266 return impl.Get()->RemoveAll(err);
1306 int32_t res = LocalSize(peekModes, err);
1324 return impl.Get()->Size(peekModes,
true, err);
1367 int32_t res = Size(peekModes, err);
1386 return impl.Get()->Size(peekModes,
false, err);
1420 impl::cache::query::QueryCursorImpl* cursorImpl = impl.Get()->QuerySql(qry, err);
1455 impl::cache::query::QueryCursorImpl* cursorImpl = impl.Get()->QueryText(qry, err);
1490 impl::cache::query::QueryCursorImpl* cursorImpl = impl.Get()->QueryScan(qry, err);
1525 impl::cache::query::QueryCursorImpl* cursorImpl = impl.Get()->QuerySqlFields(qry, err);
1571 template<
typename R,
typename P,
typename A>
1572 R
Invoke(
const K& key,
const P& processor,
const A& arg)
1576 R res = Invoke<R>(key, processor, arg, err);
1626 template<
typename R,
typename P,
typename A>
1629 typedef impl::cache::CacheEntryProcessorHolder<P, A> ProcessorHolder;
1632 ProcessorHolder procHolder(processor, arg);
1634 impl::InCacheInvokeOperation<K, ProcessorHolder> inOp(key, procHolder);
1635 impl::Out1Operation<R> outOp(res);
1637 impl.Get()->Invoke(inOp, outOp, err);
1668 template<
typename R,
typename A>
1669 R
InvokeJava(
const K& key,
const std::string& processorName,
const A& arg)
1673 R res = InvokeJava<R>(key, processorName, arg, err);
1706 template<
typename R,
typename A>
1711 impl::In3Operation<std::string, K, A> inOp(processorName, key, arg);
1712 impl::Out1Operation<R> outOp(res);
1714 impl.Get()->InvokeJava(inOp, outOp, err);
1747 using namespace impl::cache::query::continuous;
1748 using namespace common::concurrent;
1750 const SharedPointer<ContinuousQueryImpl<K, V> >& qryImpl = qry.impl;
1752 if (!qryImpl.IsValid() || !qryImpl.Get()->HasListener())
1755 "Event listener is not set for ContinuousQuery instance");
1760 ContinuousQueryHandleImpl* cqImpl = impl.Get()->QueryContinuous(qryImpl, err);
1772 template<
typename Q>
1775 const Q& initialQry)
1794 template<
typename Q>
1799 using namespace impl::cache::query::continuous;
1800 using namespace common::concurrent;
1802 const SharedPointer<ContinuousQueryImpl<K, V> >& qryImpl = qry.impl;
1804 if (!qryImpl.IsValid() || !qryImpl.Get()->HasListener())
1807 "Event listener is not set for ContinuousQuery instance");
1812 ContinuousQueryHandleImpl* cqImpl = impl.Get()->QueryContinuous(qryImpl, initialQry, err);
1830 return impl.IsValid();
1840 impl.Get()->LoadCache(err);
1857 impl.Get()->LocalLoadCache(err);
1864 common::concurrent::SharedPointer<impl::cache::CacheImpl> impl;
1869 #endif //_IGNITE_CACHE_CACHE
void LocalEvict(const std::set< K > &keys)
Attempts to evict all entries associated with keys.
Definition: cache.h:823
V GetAndRemove(const K &key)
Atomically removes the entry for a key only if currently mapped to some value.
Definition: cache.h:590
Apache Ignite API.
Definition: cache.h:48
V GetAndPutIfAbsent(const K &key, const V &val, IgniteError &err)
Stores given key-value pair in cache only if cache had no previous mapping for it.
Definition: cache.h:709
bool Replace(const K &key, const V &oldVal, const V &newVal)
Stores given key-value pair in cache only if the previous value is equal to the old value passed as a...
Definition: cache.h:782
bool PutIfAbsent(const K &key, const V &val)
Atomically associates the specified key with the given value if it is not already associated with a v...
Definition: cache.h:632
void Clear(IgniteError &err)
Clear cache.
Definition: cache.h:894
bool ContainsKeys(const std::set< K > &keys, IgniteError &err)
Check if cache contains mapping for these keys.
Definition: cache.h:215
void PutAll(Iter begin, Iter end)
Stores given key-value pairs in cache.
Definition: cache.h:479
bool Remove(const K &key)
Removes given key mapping from cache.
Definition: cache.h:1103
void PutAll(const std::map< K, V > &vals, IgniteError &err)
Stores given key-value pairs in cache.
Definition: cache.h:458
bool Replace(const K &key, const V &val, IgniteError &err)
Stores given key-value pair in cache only if there is a previous mapping for it.
Definition: cache.h:763
V LocalPeek(const K &key, int32_t peekModes, IgniteError &err)
Peeks at cached value using optional set of peek modes.
Definition: cache.h:260
query::continuous::ContinuousQueryHandle< K, V > QueryContinuous(const query::continuous::ContinuousQuery< K, V > &qry)
Start continuous query execution.
Definition: cache.h:1725
bool Remove(const K &key, IgniteError &err)
Removes given key mapping from cache.
Definition: cache.h:1130
void LoadCache()
Executes LocalLoadCache on all cache nodes.
Definition: cache.h:1836
bool IsValid() const
Check if the instance is valid.
Definition: cache.h:1828
std::map< K, V > GetAll(const std::set< K > &keys, IgniteError &err)
Retrieves values mapped to the specified keys from cache.
Definition: cache.h:355
Main entry point for all Data Grid APIs.
Definition: cache.h:68
V GetAndPutIfAbsent(const K &key, const V &val)
Stores given key-value pair in cache only if cache had no previous mapping for it.
Definition: cache.h:679
Scan query.
Definition: core/include/ignite/cache/query/query_scan.h:40
query::QueryCursor< K, V > Query(const query::TextQuery &qry)
Perform text query.
Definition: cache.h:1433
void ClearAll(const std::set< K > &keys)
Clear entries from the cache and swap storage, without notifying listeners or CacheWriters.
Definition: cache.h:940
void LocalLoadCache()
Loads state from the underlying persistent storage.
Definition: cache.h:1853
int32_t Size(int32_t peekModes)
Gets the number of all entries cached across all nodes.
Definition: cache.h:1363
V GetAndRemove(const K &key, IgniteError &err)
Atomically removes the entry for a key only if currently mapped to some value.
Definition: cache.h:610
V GetAndReplace(const K &key, const V &val, IgniteError &err)
Atomically replaces the value for a given key if and only if there is a value currently mapped by the...
Definition: cache.h:570
V GetAndPut(const K &key, const V &val, IgniteError &err)
Associates the specified value with the specified key in this cache, returning an existing value if o...
Definition: cache.h:524
int32_t Size(IgniteError &err)
Gets the number of all entries cached across all nodes.
Definition: cache.h:1349
int32_t LocalSize(IgniteError &err)
Gets the number of all entries cached on this node.
Definition: cache.h:1289
R InvokeJava(const K &key, const std::string &processorName, const A &arg, IgniteError &err)
Invokes an instance of Java class CacheEntryProcessor against the entry specified by the provided key...
Definition: cache.h:1707
void PutAll(const std::map< K, V > &vals)
Stores given key-value pairs in cache.
Definition: cache.h:439
Query cursor class template.
Definition: core/include/ignite/cache/query/query_cursor.h:54
query::QueryCursor< K, V > Query(const query::TextQuery &qry, IgniteError &err)
Perform text query.
Definition: cache.h:1453
query::QueryCursor< K, V > Query(const query::ScanQuery &qry, IgniteError &err)
Perform scan query.
Definition: cache.h:1488
query::QueryFieldsCursor Query(const query::SqlFieldsQuery &qry, IgniteError &err)
Perform sql fields query.
Definition: cache.h:1523
void GetAll(InIter begin, InIter end, OutIter dst)
Retrieves values mapped to the specified keys from cache.
Definition: cache.h:381
bool PutIfAbsent(const K &key, const V &val, IgniteError &err)
Atomically associates the specified key with the given value if it is not already associated with a v...
Definition: cache.h:654
V Get(const K &key)
Retrieves value mapped to the specified key from cache.
Definition: cache.h:284
void Put(const K &key, const V &val)
Associates the specified value with the specified key in the cache.
Definition: cache.h:403
const char * GetName() const
Get name of this cache (null for default cache).
Definition: cache.h:91
int32_t Size()
Gets the number of all entries cached across all nodes.
Definition: cache.h:1335
query::QueryFieldsCursor Query(const query::SqlFieldsQuery &qry)
Perform sql fields query.
Definition: cache.h:1503
void LocalEvict(const std::set< K > &keys, IgniteError &err)
Attempts to evict all entries associated with keys.
Definition: cache.h:843
void Put(const K &key, const V &val, IgniteError &err)
Associates the specified value with the specified key in the cache.
Definition: cache.h:423
void LocalEvict(Iter begin, Iter end)
Attempts to evict all entries associated with keys.
Definition: cache.h:862
Query fields cursor.
Definition: core/include/ignite/cache/query/query_fields_cursor.h:50
V GetAndReplace(const K &key, const V &val)
Atomically replaces the value for a given key if and only if there is a value currently mapped by the...
Definition: cache.h:547
void LocalClearAll(Iter begin, Iter end)
Clear entries from the cache and swap storage, without notifying listeners or CacheWriters.
Definition: cache.h:1077
query::continuous::ContinuousQueryHandle< K, V > QueryContinuous(const query::continuous::ContinuousQuery< K, V > &qry, const Q &initialQry, IgniteError &err)
Start continuous query execution with the initial query.
Definition: cache.h:1795
bool Remove(const K &key, const V &val, IgniteError &err)
Removes given key mapping from cache if one exists and value is equal to the passed in value.
Definition: cache.h:1171
std::map< K, V > GetAll(const std::set< K > &keys)
Retrieves values mapped to the specified keys from cache.
Definition: cache.h:331
void ClearAll(Iter begin, Iter end)
Clear entries from the cache and swap storage, without notifying listeners or CacheWriters.
Definition: cache.h:975
Cache(impl::cache::CacheImpl *impl)
Constructor.
Definition: cache.h:78
void RemoveAll()
Removes all mappings from cache.
Definition: cache.h:1246
query::continuous::ContinuousQueryHandle< K, V > QueryContinuous(const query::continuous::ContinuousQuery< K, V > &qry, const Q &initialQry)
Start continuous query execution with the initial query.
Definition: cache.h:1773
query::QueryCursor< K, V > Query(const query::SqlQuery &qry, IgniteError &err)
Perform SQL query.
Definition: cache.h:1418
static const int IGNITE_ERR_GENERIC
Generic Ignite error.
Definition: ignite_error.h:131
void Clear()
Clear cache.
Definition: cache.h:878
Text query.
Definition: query_text.h:40
void LocalClear(const K &key, IgniteError &err)
Clear entry from the cache and swap storage, without notifying listeners or CacheWriters.
Definition: cache.h:1018
R Invoke(const K &key, const P &processor, const A &arg)
Invokes an CacheEntryProcessor against the MutableCacheEntry specified by the provided key.
Definition: cache.h:1572
int32_t LocalSize(int32_t peekModes)
Gets the number of all entries cached on this node.
Definition: cache.h:1302
void LocalClearAll(const std::set< K > &keys)
Clear entries from the cache and swap storage, without notifying listeners or CacheWriters.
Definition: cache.h:1036
void RemoveAll(const std::set< K > &keys)
Removes given key mappings from cache.
Definition: cache.h:1187
Continuous query.
Definition: continuous_query.h:59
bool ContainsKey(const K &key)
Check if cache contains mapping for this key.
Definition: cache.h:137
bool ContainsKeys(const std::set< K > &keys)
Check if cache contains mapping for these keys.
Definition: cache.h:172
void RemoveAll(Iter begin, Iter end)
Removes given key mappings from cache.
Definition: cache.h:1227
query::continuous::ContinuousQueryHandle< K, V > QueryContinuous(const query::continuous::ContinuousQuery< K, V > &qry, IgniteError &err)
Start continuous query execution.
Definition: cache.h:1744
query::QueryCursor< K, V > Query(const query::SqlQuery &qry)
Perform SQL query.
Definition: cache.h:1398
bool IsEmpty()
Checks whether this cache contains no key-value mappings.
Definition: cache.h:104
void Clear(const K &key, IgniteError &err)
Clear entry from the cache and swap storage, without notifying listeners or CacheWriters.
Definition: cache.h:925
bool IsEmpty(IgniteError &err)
Checks whether this cache contains no key-value mappings.
Definition: cache.h:124
void Clear(const K &key)
Clear entry from the cache and swap storage, without notifying listeners or CacheWriters.
Definition: cache.h:907
bool Remove(const K &key, const V &val)
Removes given key mapping from cache if one exists and value is equal to the passed in value.
Definition: cache.h:1148
void RemoveAll(IgniteError &err)
Removes all mappings from cache.
Definition: cache.h:1264
R InvokeJava(const K &key, const std::string &processorName, const A &arg)
Invokes an instance of Java class CacheEntryProcessor against the entry specified by the provided key...
Definition: cache.h:1669
static void ThrowIfNeeded(const IgniteError &err)
Throw an error if code is not IGNITE_SUCCESS.
Definition: ignite_error.cpp:27
int32_t LocalSize()
Gets the number of all entries cached on this node.
Definition: cache.h:1276
bool Replace(const K &key, const V &val)
Stores given key-value pair in cache only if there is a previous mapping for it.
Definition: cache.h:736
int32_t LocalSize(int32_t peekModes, IgniteError &err)
Gets the number of all entries cached on this node.
Definition: cache.h:1322
Sql fields query.
Definition: core/include/ignite/cache/query/query_sql_fields.h:42
query::QueryCursor< K, V > Query(const query::ScanQuery &qry)
Perform scan query.
Definition: cache.h:1468
int32_t Size(int32_t peekModes, IgniteError &err)
Gets the number of all entries cached across all nodes.
Definition: cache.h:1384
V Get(const K &key, IgniteError &err)
Retrieves value mapped to the specified key from cache.
Definition: cache.h:308
bool ContainsKeys(InputIter begin, InputIter end)
Check if cache contains mapping for these keys.
Definition: cache.h:193
V LocalPeek(const K &key, int32_t peekModes)
Peeks at cached value using optional set of peek modes.
Definition: cache.h:235
Ignite error information.
Definition: ignite_error.h:94
Sql query.
Definition: query_sql.h:44
bool Replace(const K &key, const V &oldVal, const V &newVal, IgniteError &err)
Stores given key-value pair in cache only if the previous value is equal to the old value passed as a...
Definition: cache.h:806
bool ContainsKey(const K &key, IgniteError &err)
Check if cache contains mapping for this key.
Definition: cache.h:157
V GetAndPut(const K &key, const V &val)
Associates the specified value with the specified key in this cache, returning an existing value if o...
Definition: cache.h:501
void LocalClear(const K &key)
Clear entry from the cache and swap storage, without notifying listeners or CacheWriters.
Definition: cache.h:997
void LocalClearAll(const std::set< K > &keys, IgniteError &err)
Clear entries from the cache and swap storage, without notifying listeners or CacheWriters.
Definition: cache.h:1057
void RemoveAll(const std::set< K > &keys, IgniteError &err)
Removes given key mappings from cache.
Definition: cache.h:1206
void ClearAll(const std::set< K > &keys, IgniteError &err)
Clear entries from the cache and swap storage, without notifying listeners or CacheWriters.
Definition: cache.h:958
R Invoke(const K &key, const P &processor, const A &arg, IgniteError &err)
Invokes an CacheEntryProcessor against the MutableCacheEntry specified by the provided key.
Definition: cache.h:1627
@ ALL
Peeks into all available cache storages.
Definition: core/include/ignite/cache/cache_peek_mode.h:40
Continuous query handle.
Definition: core/include/ignite/cache/query/continuous/continuous_query_handle.h:40