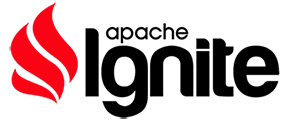 |
Apache Ignite C++
|
Go to the documentation of this file.
24 #ifndef _IGNITE_BINARY_BINARY_TYPE
25 #define _IGNITE_BINARY_BINARY_TYPE
29 #include <ignite/common/common.h>
31 #include <ignite/impl/binary/binary_type_impl.h>
37 #define IGNITE_BINARY_TYPE_START(T) \
39 struct BinaryType<T> \
46 #define IGNITE_BINARY_TYPE_END \
53 #define IGNITE_BINARY_GET_TYPE_ID_AS_CONST(id) \
54 static int32_t GetTypeId() \
63 #define IGNITE_BINARY_GET_TYPE_ID_AS_HASH(typeName) \
64 static int32_t GetTypeId() \
66 return GetBinaryStringHashCode(#typeName); \
73 #define IGNITE_BINARY_GET_TYPE_NAME_AS_IS(typeName) \
74 static void GetTypeName(std::string& dst) \
83 #define IGNITE_BINARY_GET_FIELD_ID_AS_HASH \
84 static int32_t GetFieldId(const char* name) \
86 return GetBinaryStringHashCode(name); \
93 #define IGNITE_BINARY_IS_NULL_FALSE(T) \
94 static bool IsNull(const T&) \
103 #define IGNITE_BINARY_IS_NULL_IF_NULLPTR(T) \
104 static bool IsNull(const T& obj) \
113 #define IGNITE_BINARY_GET_NULL_DEFAULT_CTOR(T) \
114 static void GetNull(T& dst) \
123 #define IGNITE_BINARY_GET_NULL_NULLPTR(T) \
124 static void GetNull(T& dst) \
164 std::string typeName;
220 template <
typename T>
233 return BinaryTypeDereferenced::GetTypeId();
243 BinaryTypeDereferenced::GetTypeName(dst);
254 return BinaryTypeDereferenced::GetFieldId(name);
265 BinaryTypeDereferenced::Write(writer, *obj);
278 BinaryTypeDereferenced::Read(reader, *dst);
289 return !obj || BinaryTypeDereferenced::IsNull(*obj);
305 #endif //_IGNITE_BINARY_BINARY_TYPE
Binary writer.
Definition: binary_writer.h:51
Apache Ignite API.
Definition: cache.h:48
static void GetNull(T *&dst)
Get NULL value for the given binary type.
Definition: binary_type.h:297
static void GetNull(T &dst)
Get NULL value for the given binary type.
Definition: binary_type.h:203
static int32_t GetFieldId(const char *name)
Get binary object field ID.
Definition: binary_type.h:252
Default implementations of BinaryType hashing functions and non-null type behaviour.
Definition: binary_type.h:213
BinaryType< T > BinaryTypeDereferenced
Actual type.
Definition: binary_type.h:224
Default implementations of BinaryType hashing functions.
Definition: binary_type.h:155
static int32_t GetTypeId()
Get binary object type ID.
Definition: binary_type.h:231
static void GetTypeName(std::string &dst)
Get binary object type name.
Definition: binary_type.h:241
Binary reader.
Definition: binary_reader.h:54
static bool IsNull(T *const &obj)
Check whether passed binary object should be interpreted as NULL.
Definition: binary_type.h:287
Default implementations of BinaryType methods for non-null type.
Definition: binary_type.h:186
static int32_t GetTypeId()
Get binary object type ID.
Definition: binary_type.h:162
IGNITE_IMPORT_EXPORT int32_t GetBinaryStringHashCode(const char *val)
Get binary string hash code.
Definition: binary_type.cpp:25
Binary type structure.
Definition: binary_type.h:149
static void Read(BinaryReader &reader, T *&dst)
Read binary object.
Definition: binary_type.h:274
static void Write(BinaryWriter &writer, T *const &obj)
Write binary object.
Definition: binary_type.h:263
static bool IsNull(const T &)
Check whether passed binary object should be interpreted as NULL.
Definition: binary_type.h:193
static int32_t GetFieldId(const char *name)
Get binary object field ID.
Definition: binary_type.h:176