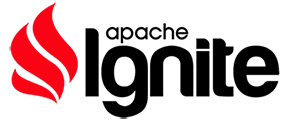 |
Apache Ignite C++
|
Go to the documentation of this file.
23 #ifndef _IGNITE_BINARY_BINARY_RAW_WRITER
24 #define _IGNITE_BINARY_BINARY_RAW_WRITER
28 #include <ignite/common/common.h>
30 #include "ignite/impl/binary/binary_writer_impl.h"
64 friend class ignite::impl::binary::BinaryUtils;
80 void WriteInt8(int8_t val);
88 void WriteInt8Array(
const int8_t* val, int32_t len);
95 void WriteBool(
bool val);
103 void WriteBoolArray(
const bool* val, int32_t len);
110 void WriteInt16(int16_t val);
118 void WriteInt16Array(
const int16_t* val, int32_t len);
125 void WriteUInt16(uint16_t val);
133 void WriteUInt16Array(
const uint16_t* val, int32_t len);
140 void WriteInt32(int32_t val);
148 void WriteInt32Array(
const int32_t* val, int32_t len);
155 void WriteInt64(int64_t val);
163 void WriteInt64Array(
const int64_t* val, int32_t len);
170 void WriteFloat(
float val);
178 void WriteFloatArray(
const float* val, int32_t len);
185 void WriteDouble(
double val);
193 void WriteDoubleArray(
const double* val, int32_t len);
200 void WriteGuid(
const Guid& val);
208 void WriteGuidArray(
const Guid* val, int32_t len);
215 void WriteDate(
const Date& val);
223 void WriteDateArray(
const Date* val, int32_t len);
230 void WriteTimestamp(
const Timestamp& val);
238 void WriteTimestampArray(
const Timestamp* val, int32_t len);
245 void WriteTime(
const Time& val);
253 void WriteTimeArray(
const Time* val, int32_t len);
260 void WriteString(
const char* val);
268 void WriteString(
const char* val, int32_t len);
277 WriteString(val.c_str(),
static_cast<int32_t
>(val.size()));
319 int32_t
id = impl->WriteArray();
356 int32_t
id = impl->WriteCollection(typ);
367 template<
typename InputIterator>
380 template<
typename InputIterator>
383 impl->WriteCollection(first, last, typ);
397 template<
typename K,
typename V>
415 template<
typename K,
typename V>
418 int32_t
id = impl->WriteMap(typ);
431 impl->WriteObject<T>(val);
444 impl->WriteEnum(val);
449 ignite::impl::binary::BinaryWriterImpl* impl;
454 #endif //_IGNITE_BINARY_BINARY_RAW_WRITER
Apache Ignite API.
Definition: cache.h:48
void WriteCollection(InputIterator first, InputIterator last)
Write values in interval [first, last).
Definition: binary_raw_writer.h:368
Binary string array writer.
Definition: binary_containers.h:48
Type
Definition: binary_consts.h:69
Time type.
Definition: time.h:35
void WriteEnum(T val)
Write binary enum entry.
Definition: binary_raw_writer.h:442
Global universally unique identifier (GUID).
Definition: guid.h:36
BinaryMapWriter< K, V > WriteMap(MapType::Type typ)
Start map write.
Definition: binary_raw_writer.h:416
@ UNDEFINED
Undefined.
Definition: binary_consts.h:74
@ UNDEFINED
Undefined.
Definition: binary_consts.h:40
void WriteCollection(InputIterator first, InputIterator last, CollectionType::Type typ)
Write values in interval [first, last).
Definition: binary_raw_writer.h:381
Binary array writer.
Definition: binary_containers.h:121
Binary collection writer.
Definition: binary_containers.h:182
BinaryCollectionWriter< T > WriteCollection(CollectionType::Type typ)
Start collection write.
Definition: binary_raw_writer.h:354
Binary raw writer.
Definition: binary_raw_writer.h:62
BinaryMapWriter< K, V > WriteMap()
Start map write.
Definition: binary_raw_writer.h:398
Binary enum entry.
Definition: binary_enum_entry.h:39
Timestamp type.
Definition: timestamp.h:37
Date type.
Definition: date.h:35
BinaryCollectionWriter< T > WriteCollection()
Start collection write.
Definition: binary_raw_writer.h:336
Binary map writer.
Definition: binary_containers.h:241
Type
Definition: binary_consts.h:35
void WriteObject(const T &val)
Write object.
Definition: binary_raw_writer.h:429
void WriteString(const std::string &val)
Write string.
Definition: binary_raw_writer.h:275
BinaryArrayWriter< T > WriteArray()
Start array write.
Definition: binary_raw_writer.h:317