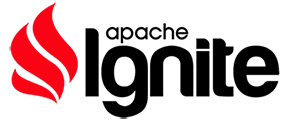 |
Apache Ignite C++
|
Go to the documentation of this file.
24 #ifndef _IGNITE_BINARY_BINARY_ENUM
25 #define _IGNITE_BINARY_BINARY_ENUM
29 #include <ignite/common/common.h>
82 return static_cast<int32_t
>(value);
92 return static_cast<T
>(ordinal);
134 template <
typename T>
147 return BinaryEnumDereferenced::GetTypeId();
157 BinaryEnumDereferenced::GetTypeName(dst);
167 return BinaryEnumDereferenced::GetOrdinal(*value);
177 return new T(BinaryEnumDereferenced::FromOrdinal(ordinal));
188 return !obj || BinaryEnumDereferenced::IsNull(*obj);
204 #endif //_IGNITE_BINARY_BINARY_ENUM
Apache Ignite API.
Definition: cache.h:48
Binary enum structure.
Definition: binary_enum.h:54
static bool IsNull(const T &val)
Check whether passed binary object should be interpreted as NULL.
Definition: binary_enum.h:107
static int32_t GetOrdinal(T *value)
Get enum type ordinal.
Definition: binary_enum.h:165
static void GetNull(T &dst)
Get NULL value for the given binary type.
Definition: binary_enum.h:117
Default implementations of BinaryEnum.
Definition: binary_enum.h:60
static int32_t GetTypeId()
Get type ID for the enum type.
Definition: binary_enum.h:67
static int32_t GetOrdinal(T value)
Get enum type ordinal.
Definition: binary_enum.h:80
Implementations of BinaryEnum nullability when INT32_MIN ordinal value used as a NULL indicator.
Definition: binary_enum.h:100
static bool IsNull(T *const &obj)
Check whether passed enum should be interpreted as NULL.
Definition: binary_enum.h:186
BinaryEnum< T > BinaryEnumDereferenced
Actual type.
Definition: binary_enum.h:138
static int32_t GetTypeId()
Get binary object type ID.
Definition: binary_enum.h:145
IGNITE_IMPORT_EXPORT int32_t GetBinaryStringHashCode(const char *val)
Get binary string hash code.
Definition: binary_type.cpp:25
static T FromOrdinal(int32_t ordinal)
Get enum value for the given ordinal value.
Definition: binary_enum.h:90
static void GetNull(T *&dst)
Get NULL value for the enum type.
Definition: binary_enum.h:196
static T * FromOrdinal(int32_t ordinal)
Get enum value for the given ordinal value.
Definition: binary_enum.h:175
Default implementations of BinaryType hashing functions and non-null type behaviour.
Definition: binary_enum.h:127
static void GetTypeName(std::string &dst)
Get binary object type name.
Definition: binary_enum.h:155